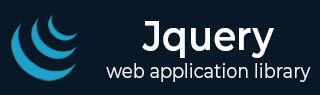
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery parentsUntil() Method
The parentsUntil() method in jQuery is used to select all ancestors siblings elements between the selector and stop. The returned elements will not include the selector and stop.
This method moves up through the parent elements and their ancestors in the DOM hierarchy until it finds a particular element, starting from a given parent element.
Note: If no parameters are specified for this method, it will return all next ancestors elements, that is similar to using the parents() method.
Syntax
Following is the syntax of parentsUntil() method in jQuery −
$(selector).parentsUntil(stop,filter)
Parameters
This method accepts the following optional parameters −
- stop (optional): A selector expression indicating the element to stop at.
- filter (optional): A string containing a selector expression to filter the results.
Example 1
In the following example, we are selecting all ancestor elements between the <span> and the <body> element −
<html> <head> <style> .ancestors * { display: block; border: 2px solid lightgrey; color: lightgrey; padding: 5px; margin: 15px; } </style> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script> <script> $(document).ready(function(){ $("span").parentsUntil("body").css({"color": "red", "border": "2px solid green"}); }); </script> </head> <body class="ancestors"> body: great-great-grandparent <div style="width:300px;">div: great-grandparent <ul>ul: grandparent <li>li: parent <span>child</span> </li> </ul> </div> </body> </html>
When we execute the above program, all the selected ancestor elements will be highlighted with red color and green background.
Example 2
In the below example, we are selecting multiple ancestors (<p>, <ul>, <div>) between <span> and the <body> element −
<html> <head> <style> .ancestors * { display: block; border: 2px solid lightgrey; color: lightgrey; padding: 5px; margin: 15px; } </style> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script> <script> $(document).ready(function(){ $("span").parentsUntil("body", "p, ul, div").css({"color": "red", "border": "2px solid green"}); }); </script> </head> <body class="ancestors"> body: great-great-great-grandparent <div style="width:300px;">div: great-great-grandparent <ul>ul: great-grandparent <li>li: grandparent <p>p: parent <span>child</span> </p> </li> </ul> </div> </body> </html>
After executing the above program, all the selected multiple ancestor elements will be highlighted with red color and green background.