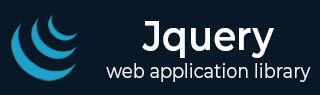
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery - nextAll( [selector] ) Method
Description
The nextAll( [selector] ) method finds all sibling elements after the current element.
Syntax
Here is the simple syntax to use this method −
selector.nextAll( [selector] )
Parameters
Here is the description of all the parameters used by this method −
selector − The optional selector can be written using CSS 1-3 selector syntax. If we supply a selector then result would be filtered out.
Example
Following is a simple example a simple showing the usage of this method −
<html> <head> <title>The jQuery Example</title> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> <script type = "text/javascript" language = "javascript"> $(document).ready(function(){ $("div:first").nextAll().addClass("hilight"); }); </script> <style> .hilight { background:yellow; } </style> </head> <body> <div>first</div> <div>sibling<div>child</div></div> <div>sibling</div> <div>sibling</div> </body> </html>
This will produce following result −
Example
Following is a simple example a simple showing the usage of this method −
<html> <head> <title>The jQuery Example</title> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> <script type = "text/javascript" language = "javascript"> $(document).ready(function(){ $("div:first").nextAll().addClass("hilight"); }); </script> <style> .hilight { background:yellow; } </style> </head> <body> <div>first</div> <div class = "hilight">sibling<div>child</div></div> <div class = "hilight">sibling</div> <div class = "hilight">sibling</div> </body> </html>
This will produce following result −
jquery-traversing.htm
Advertisements
To Continue Learning Please Login