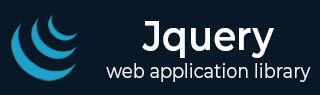
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery has() Method
The has() method selects and returns all elements that contain one or more child elements matching the specified selector.
Note: To select elements that contain multiple nested elements, separate the selectors with commas. This allows you to specify multiple criteria for the elements you want to target.
Syntax
Following is the syntax of has() method in jQuery −
$(selector).has(element)
Parameters
This method accepts the following parameter −
- element: A string containing a selector expression or an element to match elements against.
Example 1
In the following example, we are selecting all div elements that have span descendants using the has() method in jQuery −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("div").has("span").css("border", "2px solid red"); }); </script> </head> <body> <div> <p>This is a div with no spans.</p> </div> <div> <p>This is a div containing a <span>span element</span>.</p> </div> <div> <p>This is another div with no spans.</p> </div> </body> </html>
Where we execute the above program, the second div element will be selected because it has span descendants.
Example 2
Here, we are selecting all div, h2 and p elements that have a span element inside of them −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("div, h2, p").has("span").css("background-color", "green"); }); </script> </head> <body> <div>Div element <span>with span element </span>inside it.</div> <h2>Heading element <span>with span element </span>inside it.</h2> <p>Paragraph element with span element inside it.</p> <p>Paragraph element <span>with span element </span>inside it.</p> <p>Paragraph element <span>with span element </span>inside it.</p> </body> </html>
If we execute the above program, it returns the selected elements with a green background color.
Example 3
In this example, we are returning an element with multiple elements (i, span, and b) inside it −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("p").has("i, span, b").css("background-color", "yellow"); }); </script> </head> <body> <p>Paragraph element <i>with italic element </i>inside it.</p> <p>Paragraph element with <span>span </span>element inside it.</p> <p>Paragraph element with <b>bold</b> element inside it.</p> <p>Paragraph element</p> </body> </html>
If we execute the above program, it returns the selected elements with a yellow background color.