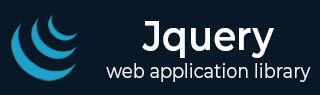
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery first() Method
The first() method in jQuery is used to select the first element of a matched set of elements. It retrieves the first element in the set of matched elements. This method is often used in combination with other jQuery methods to manipulate or retrieve specific elements within a document or a set of elements.
Note: This method allows to select the first element. If we want to select the last element, we need to use the last() method.
Syntax
Following is the syntax of first() method in jQuery −
$(selector).first()
Parameters
This method does not accept any parameters.
Example 1
In the following example, we are using the first() method to select and change the background color of the first paragraph element −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $('p').first().css("background-color", "yellow"); }); </script> </head> <body> <div> <p>This is the first paragraph.</p> <p>This is the second paragraph.</p> </div> </body> </html>
When we execute the above program, the first paragraph element in the DOM will be selected and its background color will be changed to yellow.
Example 2
In the example below, we are selecting and modifying the first p element inside the first div element −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $('div p').first().text('Since Im the first p element inside the first div element, I got modified...'); }); </script> </head> <body> <div> <p>Paragraph element 1.</p> <p>Paragraph element 2.</p> </div> <div> <p>Paragraph element 1.</p> <p>Paragraph element 2.</p> </div> </body> </html>
After executing the above program, the first p element inside the first div element will be selected, and its text will be manipulated.
Example 3
Here, we are selecting and modifying the first item in a list −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $('ul li').first().text('Since Im the first element, I got modified...'); }); </script> </head> <body> <ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> </body> </html>
If we execute the program, the first li element will be selected, and its text will be manipulated.