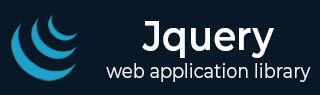
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery filter() Method
The filter method in jQuery is used to filter the elements that match a certain criteria.
This method allows you to refine a set of selected elements based on certain criteria, such as a specific CSS selector, a function that evaluates each element, or a jQuery object containing elements to match against. Elements that do not match the specified criteria are removed from the selection, and those that match will be returned.
The filter() method works opposite to the not() method.
Syntax
Following is the syntax of filter() method in jQuery −
$(selector).filter(criteria,function(index))
Parameters
This method accepts the following optional parameters −
criteria (optional): It can be a selector expression, a jQuery object, or one or more elements or an HTML snippet to be added to an existing group of elements.
function(index) (optional): A function used to run for each element in the set. This function should return true to include an element and false to exclude it.
Example 1
In the following example, we are filtering specific elements based on CSS selectors using the jQuery's filter() method −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { $('li').filter('.highlight').css('color', 'green'); }); </script> </head> <body> <ul> <li class="highlight">Item 1</li> <li>Item 2</li> <li class="highlight">Item 3</li> <li>Item 4</li> </ul> </body> </html>
When we execute the above program, the <li> elements with class "highlight" will change its color to green.
Example 2
In this example, we are demonstrating how to filter all <li> elements with class "highlight1" and id "highlight2" −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { $('li').filter('.highlight1, #highlight2').css('color', 'green'); }); </script> </head> <body> <ul> <li class="highlight1">Item 1</li> <li>Item 2</li> <li id="highlight2">Item 3</li> <li>Item 4</li> </ul> </body> </html>
After executing the above program, <li> elements with class "highlight1" and id "highlight2" will be filtered with color "green".
Example 3
The below program filters all <div> elements using the jQuery object "$otherDivs", which contains elements with the class ".other-divs" −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { // Defining a jQuery object containing elements to match against const $otherDivs = $('.other-divs'); // Filtering <div> elements using the defined jQuery object $('div').filter($otherDivs).css('border', '2px solid green'); }); </script> </head> <body> <div class="other-divs">I'm Mickey Mouse.</div> <div>I'm goofy.</div> <div class="other-divs">I'm Donald Duck.</div> <div>I'm Pluto.</div> </body> </html>
When we execute the above program, the <div> elements with class "other-divs" will have a green coloured border.
Example 4
In the below example, we are demonstrating the use of the filter() method with a function to filter <div> elements based on whether they have the class 'highlight' −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { $('div').filter(function() { return $(this).hasClass('highlight'); }).css('background-color', 'green'); }); </script> </head> <body> <div class="highlight">I'm Mickey Mouse.</div> <div>I'm goofy.</div> <div class="highlight">I'm Donald Duck.</div> <div>I'm Pluto.</div> </body> </html>
When we execute the above program, the <div> elements with class "highlight" will have a background color of green.