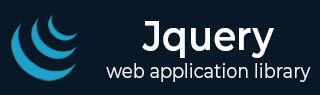
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery event.which Property
The jQuery event.which property is used to determine the specific key or button of the keyboard or mouse that was pressed during key or mouse events.
It normalizes both event.keyCode and event.charCode, but it is mostly recommended to use event.which for keyboard input.
Syntax
Following is the syntax of the jQuery event.which property −
event.which
Parameters
This method does not accept any parameter.
Return Value
This property returns the specific keyboard key or mouse button that was pressed.
Example 1
The following program demonstrates the usage of the jQuery event.which property −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> div{ color: green; font-size: 20px; font-weight: bolder; } </style> </head> <body> <p>Click on the below text.</p> <div>Tutorialspoint</div> <script> $('div').click(function(event){ alert("The pressed mouse key is: " + event.which); }) </script> </body> </html>
Output
The above program displays a p element having some text, when the mouse pointer clicks on it, a pop-up alert will appear on the browser screen displaying which mouse button you pressed −
When the mouse clicks on the displayed text −
Example 2
Following is another example of the jQuery event.which property. We use this method to retrieve which keyboard or mouse key is pressed within the input box −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> fieldset{ border: 2px solid green; padding: 10px; width: 300px; } div span{ margin: 20px 10px; } </style> </head> <body> <fieldset> <legend>MyForm</legend> Name: <input type="text" placeholder="Enter your name.."><br> <span></span> </fieldset> <script> $(document).ready(function(){ $('input').keydown(function(event){ $('span').text("Key pressed: " + event.which); }); }) </script> </body> </html>
Output
After executing the above program it displays a form that has an input, when the user starts entering something within the input the respected pressed key will be displayed next to it −