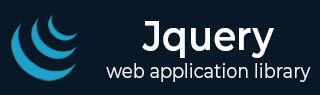
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery - trigger( event, [data] ) Method
Description
The trigger( event, [data] ) method triggers an event on every matched element.
Triggered events aren't limited to browser-based events, you can also trigger custom events registered with bind.
Syntax
Here is the simple syntax to use this method −
selector.trigger( event, [data] )
Parameters
Here is the description of all the parameters used by this method −
event − An event object or type to trigger.
data − This is an optional parameters and represents additional data to pass as arguments (after the event object) to the event handler.
Example
Following is a simple example a simple showing the usage of this method. Here you would trigger a click event on square TWO by clicking on square ONE −
<html> <head> <title>The jQuery Example</title> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> <script type = "text/javascript" language = "javascript"> $(document).ready(function() { $("#div1").click( function () { $("#div2").trigger('click'); }); $("#div2").click( function () { alert( "Square TWO is clicked"); }); }); </script> <style> div{ margin:10px;padding:12px; border:2px solid #666; width:60px;} </style> </head> <body> <span>Click square ONE to see the result:</span> <div id = "div1" style = "background-color:blue;">ONE</div> <div id = "div2" style = "background-color:blue;">TWO</div> </body> </html>
This will produce following result −
jquery-events.htm
Advertisements
To Continue Learning Please Login