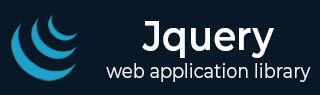
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery Event toggle() Method
The jQuery event toggle() method is used to toggle the visibility of selected elements and automatically switch between the .hide() and .show() methods within a second (default).
This method accepts an optional parameter using which you can handle the toggle speed (hide or show effect), just pass the value of the 'speed' parameter according to that it handles the toggle speed.
Syntax
Following is the syntax of the jQuery event toggle() method −
$(selector).toggle(speed, easing, callback);
Parameters
This method accepts three parameters 'speed', 'easing', and 'callback , which are described below −
- speed − It Specifies the speed of the hide or show effect (values: milliseconds, 'slow', 'fast').
- easing − It Specifies the easing function for the transition (default is 'swing').
- callback − A function to run after the toggle effect finished.
Return Value
This method does not have any return value.
Example 1
The following is the basic example of the jQuery eventĀ toggle() method −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> </head> <body> <div class="">Toggle event occured</div> <script> $('div').toggle(function(){ alert("Toggle event triggered"); }); </script> </body> </html>
Output
The above program displayed a message, and automatically hide when the toggle event triggered and a pop-up alert appear on the browser screen −
Example 2
Using an optional parameter named 'speed' to handle the speed of toggle effect −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> div{ width: 200px; padding: 10px; background-color: green; color: white; border-radius: 10px; } </style> </head> <body> <div class="">Toggle event occured</div> <span></span> <script> $('div').toggle(3000, function(){ $('span').text("Hidden"); }); </script> </body> </html>
Output
After executing the above program, a message with a green background will be displayed. It will then automatically begin to hide, and within 3 seconds, it will be completely hidden −
Example 3
Let use the "slow" and "fast" value with speed so see the toggle effect −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> div{ width: 200px; padding: 10px; background-color: green; color: white; border-radius: 10px; } .fst{ width: 200px; padding: 10px; background-color: red; color: white; border-radius: 10px; } </style> </head> <body> <p>Slow value example</p> <div class="slw">Toggle event occured(with slow value)</div> <p>Fast value example</p> <div class="fst">Toggle event occured(with slow value)</div> <span></span> <script> $('.slw').toggle((5000, "slow"), function(){ $('span').text("Hidden"); }); $('.fst').toggle((5000, "fast"), function(){ $('span').text("Hidden"); }); </script> </body> </html>
Output
Once the above program is executed, two div elements with green and red backgrounds will be displayed. The first div will be hidden within 5 seconds with a slow effect, and the second div will be hidden within 5 seconds with a fast effect −