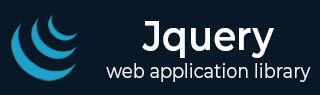
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery event.timeStamp Property
The jQuery event.timeStamp property is used to retrieve the number of miliseconds since Jan 1, 1970 upto the time the event was occured.
Why January 1, 1970, and not some other date, because January 1, 1970 is widely recognized as the "epoch date" for UNIX systems, marking the beginning of time in computing terms for these systems.
Syntax
Following is the syntax of the jQuery event.timeStamp property −
event.timeStamp
Parameters
This property does not accept any parameter.
Return Value
This property returns the number of milliseconds since Jan 1, 1970, when the event occurred.
Example 1
The following program demonstrates the usage of the jQuery event.timeStamp property −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> p{ width: 500px; padding: 10px; background-color: green; color: white; } </style> </head> <body> <p>Click on me to count the number of milliseconds since Jan 1, 1970, when the click event occurred.</p> <script> $('p').click(function(event){ alert("The click event occured number of " + event.timeStamp + " since Jan 1, 1970"); }); </script> </body> </html>
Output
The above program displayed a message, and when the user clicked on it, a pop-up alert appeared displaying the count of the number of milliseconds since January 1, 1970, when the click event occurred −
Example 2
Following is another example of the jQuery event.timeStamp property. Using this property, we are counting the number of milliseconds since January 1, 1970, when the mouseover event occurred −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> button{ width: 200px; padding: 10px; background-color: green; color: white; } </style> </head> <body> <p>Hover on the below button to count the number of milliseconds since Jan 1, 1970, when the mouseover event occurred.</p> <button>Hover on me!</button> <span></span> <script> $(document).ready(function(){ $('button').mouseover(function(event){ $('span').text("The mouseover event occured number of " + event.timeStamp + " milliseconds since Jan 1, 1970"); }); }); </script> </body> </html>
Output
After executing the program, a button will be displayed. When the mouse pointer hovers over this button, the number of milliseconds since January 1, 1970, at the time the mouseover event occurred, will be displayed next to the button −