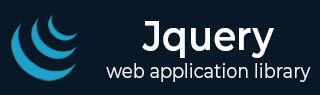
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery Event submit() Method
The jQuery event submit() method is used to bind an event handler (function) to the submit event or trigger that event on the selected element. The attach function executes when the submit event occurs.
Below are some important points about the submit event −
- The submit event occurs when the HTML forms are submitted.
- The submit event can only be used with the HTML form element.
Syntax
Following is the syntax of the jQuery event submit() method −
$(selector).submit(function);
Parameters
This method accepts an optional parameter as function, which is described below −
- function (optional) − An optional function to execute when the submit event occurs.
Return Value
This method does not return any value but attaches a function to execute when the submit event occurs.
Example 1
The following program demonstrates the usage of the jQuery event submit() method −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> </head> <body> <form> Username: <input type="text"><br><br> Password: <input type="password"><br><br> <button>Submit</button> </form> <script> $('form').submit(function(){ alert("Form submitted..."); }); </script> </body> </html>
Output
The above program displays a form with two input fields and a button. When the user submits the form, a pop-up alert will appear on the browser screen −
Example 2
Form validation using 'submit' event
Following is another example of the jQuery event submit() method. We use this method to prevent the form submission until the form is validated −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> </head> <body> <form> Username: <input type="text"><br><br> Password: <input type="password"><br><br> <button>Submit</button> <span></span> </form> <script> $('form').submit(function(event){ if(!isValidForm()){ event.preventDefault(); } }); function isValidForm(){ var $uname = $('input[type="text"]').val(); var $psw = $('input[type="password"]').val(); if($uname.trim().length == 0){ $('span').text("Username is required...!"); $('span').css("color", "red"); return false; } else if($psw.trim().length == 0){ $('span').text("Password is required...!"); $('span').css("color", "red"); return false; } else{ $('span').text("Form submitted...!"); $('span').css("color", "green") return true; } } </script> </body> </html>
Output
After executing the above program, it will display a form with two input fields and a button. If the input fields are empty, the form cannot be submitted, and an error message will be displayed in "red" color −