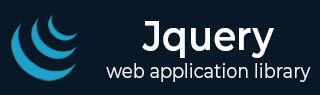
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery event.namespace Property
The jQuery event.namespace property is used to specify the namespace when the event was triggered. It returns the specified or custom namespace when the event triggers.
In JQuery, a namespace is an object that encapsulates functions, variables, and other objects. It provides a way to organize and group related code and prevent naming conflicts.
Syntax
Following is the syntax of the jQuery event.namespace property −
event.namespace
Parameters
- This method does not accept any parameter.
Return Value
This property returns the custom namespace when the event is triggered.
Example 1
Binding an event with namespace.
The following is the basic example of the jQuery event.namespace property −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <style> p{ font-size: 20px; color: green; font-weight: bold; } </style> </head> <body> <p>Click on me</p> <script> $("p").on("click.TutorialsPoint", function(event) { alert("Event namespace is: " + event.namespace); }); </script> </body> </html>
Output
The program above displays a button. When the button is clicked, it shows a 'undefined' as namespace, till the event not get triggered it will return undefined −
When the button is clicked −
Example 2
Triggering an event with a namespace.
The following is another example of the jQuery event.namespace property. We use this method to specify and retrieve the namespace when event was triggered −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <style> div{ width: 200px; padding: 10px; background-color: green; color: white; } </style> </head> <body> <p>Mouse enter on the below box</p> <div>TutorialsPoint</div><br> <button>Remove</button><br> <span></span> <script> $("div").on("mouseenter.TutorialsPoint", function(event) { $('span').text("Event namespace is: " + event.namespace); $('span').css("color", "green"); }); $("div").on("mouseenter", function(){ $(this).trigger("mouseenter.TutorialsPoint"); }) $('button').click(function(){ $('div').off("mouseenter.TutorialsPoint"); $('span').text("Removed...!"); $('span').css("color", "red"); }); </script> </body> </html>
Output
After executing the above program it displayed a <div> element and a button, when mousepointer enters "div" element namepaces will be displayed, when button is clicked the namespace is removed −