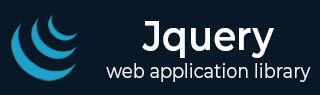
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery Event mouseout() Method
The jQuery event mouseout() method attaches an event handler to the mouseout event. It triggers when the mouse pointer leaves the selected element or any of its children's elements.
It is different from the jQuery mouseleave event, which is only triggered when the mouse pointer leaves the selected element itself.
Syntax
Following is the syntax of the jQuery event mouseout() method −
$(selector).mouseout(function)
Parameters
This method accepts an optional parameter as a function, which is described below −
- function − An optional function to execute when the mouseout event triggers.
Return Value
This method does not return any value, instead binds an event handler to the mouseout event.
Example 1
The following example demonstrates the usage of the jQuery event mouseout() method −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> p{ background-color: green; padding: 10px; color: white; width: 300px; } </style> </head> <body> <p>Enter and leave the mouse pointer on me</p> <script> $('p').mouseout(function(){ alert("The mouse pointer leaves the selected element.") }); </script> </body> </html>
Output
The above program displayed a message, when the mouse pointer leaves the displayed message, a pop-up alert message will appear on the browser screen −
When the mouse pointer leaves the selected element −
Example 2
The jQuery event mouseout() method can handle mouse interactions between a parent element and its child −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> div{ width: 350px; padding: 20px; background-color: aqua; } p{ background-color: green; padding: 10px; width: 300px; } </style> </head> <body> <div class="parent"> Parent <p class="child">Child</p> </div> <script> $(document).ready(function() { $('.parent').mouseout(function() { $(this).css('background-color', 'lightgreen'); }); $('.child').mouseout(function() { $(this).css('color', 'white'); }); }); </script> </body> </html>
Output
In the above program, theĀ mouseoutĀ event is attached to both (parent and child elements). When the mouse pointer leaves the parent element, its background color changes to 'light green'. Similarly, when the mouse pointer leaves the child element, its text color changes to 'white' −
Example 3
In the example below, we are using the jQuery event mouseout() method to change the style and print the entered text of the input field when the mouse moves out from the input element −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> </head> <body> <p>To change the input field style, simply move your mouse pointer away from the input field below.</p> <label for="">Name: </label> <input type="text"><br><br> <span></span> <script> $('input').mouseout(function(){ $(this).css( { "background-color": "lightgreen", "color": "white", "width": "300px", "border-radius" : "10px", "transition": "1s", "padding": "10px" }); $value = $('input').val(); $('span').text("Input data: " + $value); }); </script> </body> </html>
Output
After executing the program, it displays an input field. When the mouse leaves the input field, the background changes to "green", and the entered text is displayed next to the input field −