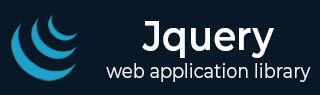
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery Event mousemove() Method
The jQuery event mousemove() method is used to bind an event handler to the mousemove event or to trigger that event on the selected element. It occurs when the mouse pointer moves within the selected element.
Syntax
Following is the syntax of the jQuery event mousemove() method −
$(selector).mousemove(function)
Parameters
This method accepts an optional parameter as a function, which is described below −
- function − An optional function to execute when the mousemove event occurs.
Return Value
This method does not have any return value.
Example 1
The following is the basic example of the jQuery event mousemove() method −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> div{ width: 300px; padding: 20px; background-color: green; color: white; } </style> </head> <body> <div>Move the mouse pointer within this box</div> <script> $('div').mousemove(function(){ alert("Mouse pointer moved"); }); </script> </body> </html>
Output
The above program displayed a box, when the mouse pointer moved within the box a pop-up alert message will appear on the browser screen −
When the mouse pointer moved within the displayed box −
Example 2
In the example below, we change the style of the 'p' element when the mouse moves within it −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> </head> <body> <p>Mouse move to change style</p> <script> $('p').mousemove(function(){ $(this).css({ 'color' : 'green', 'font-style':'italic', 'font-size': '30px', 'font-weight': 'bold' }); }); </script> </body> </html>
Output
Once the above program is executed, a text is displayed, the mouse moves within the text, and the style of the displayed text will be changed −
Example 3
Let's see another scenario of the jQuery event mousemove() method. Using this method, we are trying to track the mouse pointer position −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> span{ width: 300px; height: 50px; background-color: aqua; padding: 20px; } </style> </head> <body> <p>Move your mouse pointer and see the pointer position</p><br> <span>X: 0, Y: 0 </span> <script> $(document).mousemove(function(){ $("span").text("X: " + event.pageX + ", Y: " + event.pageY); }); </script> </body> </html>
Output
After executing the above program, it displays a box. When the mouse pointer moves over the browser screen, the position of the pointer will be displayed within the box itself −