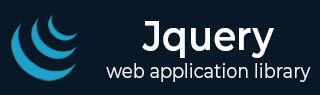
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery Event mouseenter() Method
The jQuery event mouseenter() method attaches an event handler to the mouseenter event or occurs when the mouse pointer enters on the selected elements.
The mouseenter() method is similar to the mouseover() method; the difference between these is that the mouseover event bubbles up, whereas the mouseenter event does not.
Syntax
Following is the syntax of the jQuery event mouseenter() method −
$(selector).mouseeneter(function)
Parameters
This method accepts an optional parameter as a function, which is described below −
- function − An optional function to execute when the mouseeneter event occurs.
Return Value
This method does not return any value but attaches an event handler to the mouseeneter event.
Example 1
Following is the basic example of the jQuery event mouseenter() method −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> </head> <body> <p>Mouse pointer enter on me!</p> <script> $('p').mouseenter(function(){ alert("Mouse pointer enter on the selected element..!"); }); </script> </body> </html>
Output
The above program displays a message when the mouse pointer enters (or hovers) on the displayed message a pop-up alert will appear on the browser screen −
When the mouse pointer enters on the selected element −
Example 2
The following is another example of the jQuery event mouseenter() method. Here, we change the background color of the input field when the mouse pointer enters (or hovers) on input field −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> </head> <body> <p>Hovers the mouse pointer on the below input field</p> Name: <input type="text" placeholder="Name"> <script> $('input').mouseenter(function(){ $(this).css({"background-color": "green", "color": "white"}); }); </script> </body> </html>
Output
After executing the above program, an input field will be displayed when mouse pointer enters on the input element −
When mouse pointer enters on an input field −
Example 3
Let's see another scenario of the jQuery event mouseenter() method. Here, we combine the mouseenter and mouseover events to toggle the styles of the element −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> .enter{ width: 250px; background-color: green; color: white; border-radius: 5px; padding: 10px; transition: 1s; } .leave{ width: 200px; background-color: rgb(63, 77, 63); color: white; border-radius: 10px; transition: 0.5s; padding: 12px; } </style> </head> <body> <p>Enter and leave mouse pointer on button to toggle the styles</p> <button>Toggle my Styles</button> <script> $('button').mouseenter(function(){ $(this).addClass('enter'); }).mouseleave(function(){ $(this).addClass('leave'); }); </script> </body> </html>
Output
Once the above program is executed, it displays a button, when the mouse pointer enters the button element, the background changes to 'green', and when leaves background changes to 'black' −