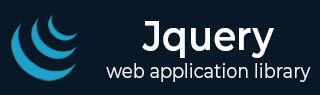
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery event.delegateTarget Property
The jQuery event.delegateTarget property refers to the element to which an event handler is attached or returns the element where the currently called event handler was attached.
This property is useful in the context of event delegation, where an event handler is attached to a parent element to be processed.
The event.delegateTarget is equivalent to the event.currentTarget property when the event is directly attached to an element, and no delegation occurs.
Syntax
Following is the syntax of the jQuery event.delegateTarget property −
event.delegateTarget
Parameters
This method does not accept any parameter.
Return Value
This property returns the element where the currently called event handler was attached.
Example 1
The program below demonstrates how to use the jQuery event.delegateTarget property −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <style> p{ font-size: 30px; font-weight: bold; } </style> </head> <body> <div> <p>Click me to change color</p> </div> <div> <p>Click me to change color</p> </div> <script> $('p').on("click", function(event){ $(event.delegateTarget).css("color", "green"); }) </script> </body> </html>
Output
After executing the above program, two "p" elements will be displayed, each containing some text. When the user clicks on an individual "p" element, the color of that specific element will change −
Example 2
The following is another example of the jQuery event.delegateTarget property. We use this property to retrieve the element to which an event handler is currently attached −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <style> p{ font-size: 20px; font-weight: bold; } div{ width: 200px; padding: 5px; border-radius: 10px; } </style> </head> <body> <div> <p>Inside first div</p> <button>Click me</button> </div> <div> <p>Inside second div</p> <button>Click me</button> </div> <script> $('div').on("click", "button", function(event){ $value = $(this).closest('div'); alert($value.find('p').text()); $(event.delegateTarget).css({"background-color": "green", "color": "white"}); }) </script> </body> </html>
Output
The above program displays two div elements, each containing a "p" and a "button" element. When the button is clicked, the background color of the respective div element will change to green −