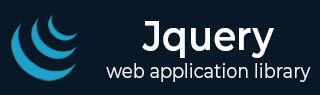
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery Event dblclick() Method
The jQuery event dblclick() method is used to trigger an action when a dblclick event occurs on a selected element.
You can use this method to either trigger the dblclick (double-click) event or attach a function that will execute when an event occurs. This method also can be used to bind the handler with an event or trigger on the specified element.
Syntax
Following is the syntax of the jQuery event dblclick() method −
$(selector).dblclick(function)
Parameters
This method accepts an optional parameter as 'function', which is described below −
- function (optional) − A function to run (execute) when the dblclick event occurs.
Return Value
This method does not return any value but binds a handler with an event.
Example 1
The following program demonstrates the usage of the jQuery event dblclick() method −
<html> <head> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> <style> .demo{ background-color: green; padding: 10px; width: 200px; color: white; } </style> </head> <body> <p>Double-click on the below div element to trigger an event.</p> <div class="demo"> Double-click on me! </div> <script> $('div').dblclick(function(){ alert("dblclick event ouccured"); }); </script> </body> </html>
Output
The above program displayed a message, once we double-clicked on that message, an alert pop-up message will appear on your browser screen −
When the user double-clicks on the displayed message −
Example 2
The following is another example of the jQuery event dblclick() method. We use this method to trigger an action (performing addition operation), whenever the user double-clicks on the selected element that is button −
<html> <head> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> </head> <body> <span></span> <p></p> <button>Double click to find sum</button> <script> let a = Number(10); let b = Number(20); document.querySelector('span').innerHTML = "a = " + a + ", b = " + b; $('button').dblclick(function(){ document.querySelector('p').innerHTML = "Sum is: " + (a + b); }); </script> </body> </html>
Output
After executing the program, it displays two variables, a and b, with respective values of 10 and 20. When the user double-clicks on the button, the sum of these variables will be displayed −
When the user double-clicks on the displayed button −
Example 3
In the example below, we use the jQuery event dblclick() method to bind a handler with an event, when the user double-clicks on the specified element the "background color" of that element will be changed −
<html> <head> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> <style> div{ width: 300px; padding: 10px; text-align: center; background-color: rgb(84, 182, 150); color: white; } </style> </head> <body> <div class="change">Double click to change background color</div> <script> $('div').dblclick(function(){ $(this).css("background-color", "blue"); }); </script> </body> </html>
Output
After executing the program, it displays a message with a background color of red. When the user double-clicks on this message, the background color will change to blue −
When the user double-clicks on the displayed message −
Example 4
Using the jQuery dblclick() event method, we are trying to trigger an action to hide a specified element when the user double-clicks on the displayed message −
<html> <head> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> <style> h2{ background-color: aqua; padding: 10px; width: 300px; } </style> </head> <body> <h2>Double click to hide me!</h2> <script> $('h2').dblclick(function(){ $(this).hide(); }); </script> </body> </html>
Output
The above program displayed a message, when a user double-clicks on it, it will be hidden −
When the user double-clicks on the displayed message −