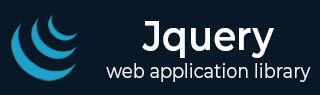
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery event.data Property
The jQuery event.data property retrieves optional data passed to an event method when the current execution handler is bound.
You can include an optional data object when binding an event handler to the jQuery on() method. This data can be anything you want to pass to the event handler function, such as strings, numbers, or objects, and during the execution of the event, this data is accessible using the event.data property.
Syntax
Following is the syntax of the jQuery event.data property −
event.data
Parameters
This method does not accept any parameter.
Return Value
This method returns the passed data value to the event handler function.
Example 1
The following program demonstrates the usage of the jQuery event.data property −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> </head> <body> <p>Mouse hover on me</p> <script> $('p').on("mouseenter", {msg: "TutorialsPoint"}, function(event){ alert("Passed data: " + event.data.msg); }); </script> </body> </html>
Output
The above program displays a message, and when the mouse pointer hovers over it, a pop-up alert appears on the browser screen showing the data value that was passed to the event handler −
When the mouse pointer enters over the displayed message −
Example 2
In the example below, we use the jQuery event.data property to retrieve the passed multiple data to the event handler function −
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7/jquery.min.js"></script> <style> button{ width: 100px; padding: 10px; cursor: pointer; background-color: green; color: white; } span{ font-size: 20px; color: green; font-weight: bold; } </style> </head> <body> <p>Click on the 'Display' button to print the passed data.</p> <button>Display</button> <span></span> <script> $('button').on("click", {name: "Rahul", age: 24, city: "Hyderabad"}, function(event){ $('span').text( "Name: " + event.data.name+ ' | ' + "Age: " + event.data.age + ' | ' + "City: " + event.data.city ) }); </script> </body> </html>
Output
After executing the above program, a button will be displayed, and when it is clicked, the data passed will be printed next to it −