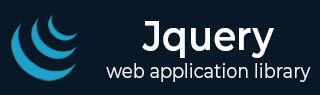
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery Event click() Method
The jQuery event click() method is used to quickly attach a click handler to selected elements. When the click() method is invoked on an element, it either triggers the click event or, if a function is provided, it attaches that function to execute when the click event occurs.
Syntax
Following is the syntax of the jQuery event click() method −
$(selector).click(function)
Parameters
This method accepts an optional parameter as 'function', which is described below −
- function (optional) − An optional parameter that specifies the function to run when the click event occurs.
Return Value
This method attaches a click handler to selected elements but does not return a value.
Example 1
The following program demonstrates the usage of the jQuery event click() method −
<html> <head> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> </head> <body> <p>Click on the below button to see a pop-up alert message.</p> <button>Click me!</button> <script> $('button').click(function(){ alert("You clicked on button"); }); </script> </body> </html>
Output
Once the above program is executed, a button will be displayed on the browser screen. If the user clicks on the button a pop-up alert will be triggered −
Example 2
In the following example, we use the jQuery click() method to attach a click handler to a specified div element. When the user clicks on the "div" element, a pop-up alert message will be displayed on the browser screen −
<html> <head> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> </head> <body> <div>Click on me!</div> <span></span> <script> $('div').click(function(){ alert("You clicked on div element."); }); </script> </body> </html>
Output
In the above program, when the user clicks on the displayed message, a pop-up alert will appear on the browser screen −
Example 3
In the example below, we attach a click handler to the button element using the jQuery click() method. When the button is clicked, a message will appear next to it −
<html> <head> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> </head> <body> <p>Click on the below button to print some message</p> <button>Click me!</button> <span></span> <script> $('button').click(function(){ document.querySelector('span').innerHTML = "Welcome to TutorialsPoint....!"; }); </script> </body> </html>
Output
Before clicking on the button −
Once you click on the button −