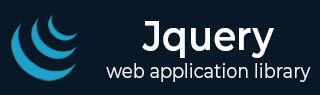
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery Effect slideUp() Method
The slideUp() method in jQuery method is used to slides-up (or hides) the selected elements by gradually reducing their height to zero. It creates a sliding animation upwards, making the element disappear from the DOM.
This effect is mostly used in the scenarios such as creating dropdown menus or hiding elements from the DOM after clicking them, etc.
To slide-down (or show) the selected elements, we need to use the slideDown() method.
Syntax
Following is the syntax of jQuery slideUp() method −
$(selector).slideUp(speed,easing,callback)
Parameters
This method accepts the following parameters −
speed (optional): A string or number determining how long the animation will run. Default value is 400 milliseconds. Possible values are: milliseconds, slow, fast.
easing (optional): A string indicating which easing function to use for the transition. Default value is "swing". Possible values are: swing, linear.
callback (optional): A function to be executed once the animation is complete.
Example 1
In this example, we are using the slideUp() method on the <div> element with the ID "box", causing it to slide up and disappear gradually −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("#slideUpButton").click(function(){ $("#box").slideUp(); }); }); </script> </head> <body> <div id="box" style="width: 200px; height: 200px; background-color: lightblue;"> <p>This is a box.</p> </div> <button id="slideUpButton">Slide Up</button> </body> </html>
When we click the button, it triggers the slideUp() and the <div> slides up gradually and will be hidden.
Example 2
In the below example, we are using the slideUp() method with custom speed (5000; i.e. 5 seconds) −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("#slideUpButton").click(function(){ $("#box").slideUp(5000); // Slide up over 5 seconds }); }); </script> </head> <body> <div id="box" style="width: 200px; height: 200px; background-color: lightblue;"> <p>This is a box.</p> </div> <button id="slideUpButton">Slide Up</button> </body> </html>
When we click the "Slide Up" button, it triggers the slideUp() method on the <div> element with the ID "box", and the animation takes 5 seconds to complete.
Example 3
In this example, we have provided a callback function as the second argument to slideUp() method −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $("#slideUpButton").click(function(){ $("#box").slideUp(1000, function(){ alert("The box has slid up!"); }); }); }); </script> </head> <body> <div id="box" style="width: 200px; height: 200px; background-color: lightblue;"> <p>This is a box.</p> </div> <button id="slideUpButton">Slide Up</button> </body> </html>
The callback function will be executed once the animation is complete, and it displays an alert message saying "The box has slid up!".