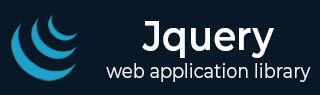
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
slideDown( speed, [callback] ) Method
Description
The slideDown() method reveals all matched elements by adjusting their height and firing an optional callback after completion.
Syntax
Here is the simple syntax to use this method −
selector.slideDown( speed, [callback] );
Parameters
Here is the description of all the parameters used by this method −
speed − A string representing one of the three predefined speeds ("slow", "normal", or "fast") or the number of milliseconds to run the animation (e.g. 1000).
callback − This is optional parameter representing a function to call once the animation is complete.
Example
Following is a simple example a simple showing the usage of this method −
<html> <head> <title>The jQuery Example</title> <script type = "text/javascript" src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"> </script> <script type = "text/javascript" language = "javascript"> $(document).ready(function() { $("#down").click(function(){ $(".target").slideDown( 'slow', function(){ $(".log").text('Slide Down Transition Complete'); }); }); $("#up").click(function(){ $(".target").slideUp( 'slow', function(){ $(".log").text('Slide Up Transition Complete'); }); }); }); </script> <style> p {background-color:#bca; width:200px; border:1px solid green;} </style> </head> <body> <p>Click on any of the buttons</p> <button id = "up"> Slide Up </button> <button id = "down"> Slide Down</button> <div class = "target"> <img src = "../images/jquery.jpg" alt = "jQuery" /> </div> <div class = "log"></div> </body> </html>
This will produce following result −
jquery-effects.htm
Advertisements
To Continue Learning Please Login