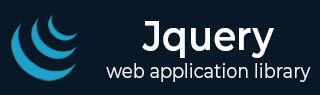
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Selectors
- jQuery - Events
- jQuery - Effects
- jQuery - HTML/CSS
- jQuery - Traversing
- jQuery - Miscellaneous
- jQuery - Properties
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery Effect fadeIn() Method
The fade-in effect is a visual transition where an element gradually becomes more visible from being initially hidden or transparent. It smoothly increases the opacity of the element over a specified duration, creating a smooth transition.
The fadeIn() method is used in jQuery to make a hidden element visible by fading them in over a specified duration. It animates the opacity of the selected elements from 0 to 1, making them visible.
This method can also be used together with "fadeOut()" method.
Syntax
Following is the syntax of fadeIn() method in jQuery −
$(selector).fadeIn(speed,easing,callback)
Parameters
This method accepts the following optional parameters −
speed (optional): A string or number determining how long the animation will run. It can take values like "slow", "fast", or a specific duration in milliseconds (e.g., 1000 for 1 second).
easing (optional): A string specifying the easing function to use for the animation (e.g., "swing" or "linear").
callback (optional): A function to call once the animation is complete. It's executed for each selected element.
Example 1
In the following example, we are using the JavaScript fadeIn() method to add a "slow" fade in effect on a <div> element −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { $("#fadeButton").click(function() { $("#content").fadeIn("slow"); }); }); </script> </head> <body> <button id="fadeButton">Click me!</button> <div id="content" style="display: none;"> <h2>Welcome to Tutorialspoint</h2> <p>This text will fade in when the button is clicked.</p> </div> </body> </html>
If we click on the button, the <div> element will fade in.
Example 2
In this example, the <div> element automatically fades in when the page loads −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { $("#content").fadeIn("slow"); }); </script> </head> <body> <div id="content" style="display: none;"> <h2>Welcome to Tutorialspoint!</h2> <p>This content fades in automatically when the page loads.</p> </div> </body> </html>
If we execute the above program, the content of <div> element will fade in automatically.
Example 3
The following example fades in multiple elements one after the other −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { $("#fadeButton").click(function() { $(".content").each(function(index) { $(this).delay(500 * index).fadeIn("slow"); }); }); }); </script> </head> <body> <button id="fadeButton">Click me!</button> <div class="content" style="display: none;"> <h2>First Element</h2> </div> <div class="content" style="display: none;"> <h2>Second Element</h2> </div> <div class="content" style="display: none;"> <h2>Third Element</h2> </div> </body> </html>
If we click on the button, all the three <div> elements will fade in.
Example 4
In the below example, we are using both fadeIn() and fadeOut() methods together to create a simple toggle effect −
<html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { $("#toggleButton").click(function() { $("#content").fadeOut("slow", function() { // Fade out complete callback $("#content").fadeIn("slow"); }); }); }); </script> </head> <body> <button id="toggleButton">Click me!</button> <div id="content"> <h2>Welcome to Tutorialspoint!</h2> </div> </body> </html>
The <div> element will fade out and then fade back in when the button is clicked.