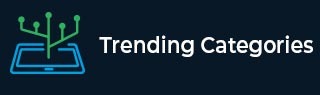
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
JODA- Time
JODA- Time is an API developed by joda.org which provides better classes and more effective methods for handling date and time than classes from java. util package like calendar, Gregorian calendar, date, etc. It is included in Java 8.0 with the java.time package.
To include, we need to import following −
import java.time.*;
Basic Features of JODA- Time
Some of the features of JODA- Time are mentioned below −
Simple field accessors are used, such as getYear(), getDayofWeek(), and getDayofYear()
It supports seven different calendar systems including, Julian, Islamic, Gregorian, Coptic, Ethiopic, Buddhist and Gregorian-Julian.
Option to develop our own calendar is provided
It offers a wide range of methods for calculating time and dates
Time zones are used from the database. This database was personally updated many times in a year.
Its methods execute more quickly than prior Java 7.0 methods
Providing superior performance
Its object cannot be changed
Thread safe
Important Classes in java.time Package
DateTime
Syntax
DateTime dt= new DateTime();
Explanation
Creates a datetime object representing the current date and time in milliseconds as determined by the system clock. It is developed using the ISO Calendar in the default time zone. Immutable replacement for JDK Calendar
LocalDate
Syntax
LocalDate today = LocalDate.now() //gives System date into LocalDate object using now method. System.out.println(today) // 2018-08-03 int d = today.getDayOfMonth(); // 03
Explanation
This class represents a date in the form of year-month-day and useful for representing a date without time and time zone.
LocalTime
Syntax
// get current date and time LocalDateTime dt = LocalTime.now(); System.out.println("%s", dt);
Explanation
It handles both date and time without considering the time zone.
Setting Up Environment
In eclipse, create your Java Project
Extract the contents of the most recent JodaTime.tar.gz file by clicking here to get it.
Find your project in eclipse’s package explorer, right click on it, and then choose call project. Folder>New>Libs
Joda-time-2.1.jar should be copied or dragged into the newly established libs folder
Once more in a package explorer, right click on your project, then select properties> java build path>libraries>add jars>joda-time-2.1.jar
Now you might test with this code − -
DateTime test = new DateTime();
Basic Example
// Java program to illustrate // functions of JODA time import org.joda.time.DateTime; import org.joda.time.LocalDateTime; public class JodaTime { public static void main(String[] args) { DateTime now = new DateTime(); System.out.println("Current Day: " + now.dayOfWeek().getAsText()); System.out.println("Current Month: " + now.monthOfYear().getAsText()); System.out.println("Current Year: " + now.year().getAsText()); System.out.println("Current Year is Leap Year: " + now.year().isLeap()); // get current date and time LocalDateTime dt = LocalDateTime.now(); System.out.println(dt); } }
Output
Current Day: Monday Current Month: April Current Year: 2023 Current Year is Leap Year: false 2023-04-06T13:12:16.672
Advantages
Joda time has many advantages, some of them are mentioned below −
Using Java in a similar way on several platforms
Supports more calendars, including, Ethiopic, and Buddhist
Improved performance
Simple interoperability − Internally, the library employs a millisecond instant, the same unit of time used by the JDK and other widely used time representations. Because of this, interoperability is simple, and Joda-Time has built-in JDK compatibility.
Disadvantages
Requires installation of package
Requires updates from Joda.org
Why use JODA- Time?
Before Java 8, The date/time API presented multiple design problems. Among the issues is the fact that the date and SimpleDateFormatter classes are not thread safe.
To address the issue, Joda time uses immutable classes for handling date and time. The date class doesn’t represent an actual date. But instead, it specifies an instant in time with millisecond precision. Joda time offers a clean and fluent API for handling dates and time.
Conclusion
Joda time is the widely used date and time processing library. Before the release of Java 8, its purpose was to provide an intuitive API for processing date and time and also address the time design issue that existed in the java date/time API. It requires the installation of packages and updates from Joda.org. It has many advantages. It supports many different types of calendars like Julian, Islamic, Gregorian, Coptic, Ethiopic, Buddhist and Gregorian-Julian. It has many basic features which help developers to use this in their application.