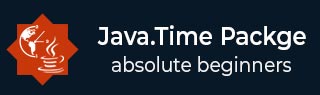
- java.time Package Classes
- java.time - Home
- java.time - Clock
- java.time - Duration
- java.time - Instant
- java.time - LocalDate
- java.time - LocalDateTime
- java.time - LocalTime
- java.time - MonthDay
- java.time - OffsetDateTime
- java.time - OffsetTime
- java.time - Period
- java.time - Year
- java.time - YearMonth
- java.time - ZonedDateTime
- java.time - ZoneId
- java.time - ZoneOffset
- java.time Package Enums
- java.time - Month
- java.time Useful Resources
- java.time - Discussion
java.time.Clock.tick() Method Example
Description
The java.time.Clock.tick() method obtains a clock that returns instants from the specified clock truncated to the nearest occurrence of the specified duration.
Declaration
Following is the declaration for java.time.Clock.tick() method.
public static Clock tick(Clock baseClock, Duration tickDuration)
Parameters
baseClock − the base clock to add the duration to, not null.
tickDuration − the duration of each visible tick, not negative, not null.
Return Value
a clock that ticks in whole units of the duration, not null.
Exception
IllegalArgumentException − if the duration is negative, or has a part smaller than a whole millisecond such that the whole duration is not divisible into one second.
ArithmeticException − if the duration is too large to be represented as nanos.
Example
The following example shows the usage of java.time.Clock.tick() method.
package com.tutorialspoint; import java.time.Clock; import java.time.Duration; public class ClockDemo { public static void main(String[] args) { Clock clock = Clock.systemUTC(); Duration tickDuration = Duration.ofNanos(250000); Clock clock1 = Clock.tick(clock, tickDuration); System.out.println("Clock : " + clock.instant()); System.out.println("Clock1 : " + clock1.instant()); } }
Let us compile and run the above program, this will produce the following result −
Clock : 2017-03-07T06:53:24.870Z Clock1 : 2017-03-07T06:53:24.951Z
To Continue Learning Please Login