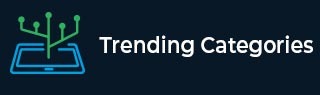
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
JavaScript String Prototype Property
In JavaScript, every object has its own properties, and each object contains the prototype property. The string is also an object in JavaScript. So, it also contains the prototype property.
The prototype property is nested in the object, meaning every prototype property contains another prototype property. The string object’s prototype property contains the default methods and properties. However, developers can customize the prototype property and add methods and properties to the string prototype.
In this tutorial, we will learn to use the methods of string prototype property and customize them.
Syntax
Users can follow the syntax below to add any method to the string prototype property.
String.prototype.method_name = function () { // function code };
In the above syntax, method_name should be the name of the method which we want to add to the string prototype.
Example 1 (Using toUpperCase() and toLowerCase() Methods)
In the example below, we have used the toUpperCase() and toLowerCase() methods of the string prototype property, which converts the string into uppercase and lowercase, respectively.
In the output, users can observe the resultant strings.
<html> <body> <h3> Using the <i> toUpperCase() and toLowerCase() </i> methods of string prototype property to customize the strings </h3> <div id = "output"> </div> <script> let output = document.getElementById("output"); var str = "Hello readers! This string contains uppercase and lowerCase letters."; var res = str.toUpperCase(); var res1 = str.toLowerCase(); output.innerHTML = "Original string: " + str + "<br>" + "Uppercase string: " + res + "<br>" + "Lowercase string: " + res1; </script> </body> </html>
Example 2 (Using the Length Property)
In the example below, we have demonstrated to use of the default properties of the string prototype property. Here, we have used the ‘length’ property to count the total number of characters in the string.
In the output, we can see the total character or length of the string, which we have calculated using the length property.
<html> <body> <h3> Using the <i> length </i> property of the string prototype property to get the length of the string </h3> <div id = "output"> </div> <script> let output = document.getElementById("output"); let string = "JavaScript string contains prototype property."; let len = string.length;; output.innerHTML = "The original string is: " + string + "<br><br>"; output.innerHTML += "The length of the string is: " + len; </script> </body> </html>
Example 3 (Adding Method to String Prototype)
We can also add custom methods to the string prototype property. Here, we have added the countWords() property to the string prototype, which counts the total number of words in the string and returns its value.
Here, we have split the string by using the “ “ as a delimiter and counted the length of the resultant array to count the total number of words in the string. In the code, we can see that we can execute the countWords() method by taking any string as a reference as, like other string methods.
<html> <body> <h3> Adding the <i> countWords() method to the </i> string prototype property </h3> <div id = "output"> </div> <script> let output = document.getElementById("output"); // adding countWords() method to string prototype property. String.prototype.countWords = function () { return this.split(" ").length; }; let string1 = "This is a string"; let string2 = "Hey, do you know how many words are there in this string?"; output.innerHTML = "The number of words in the string '" + string1 + "' is " + string1.countWords() + "<br>"; output.innerHTML += "The number of words in the string '" + string2 + "' is " + string2.countWords(); </script> </body> </html>
Example 4 (Customizing Default Methods of String Prototype)
In this example, we have demonstrated how we can customize the default methods of the string prototype. Here, we have customized the toUpperCase() method. Generally, the toUpperCase() method returns the string after converting all string characters into uppercase.
We have customized it to return a string after converting only the first character in the uppercase. Here, we return the same string if the first character is already in uppercase. Otherwise, we use ASCII value to convert the first character to uppercase.
In the output, we can see that the toUpperCase() method converts only the first character of the string in uppercase rather than the whole string in uppercase.
<html> <body> <h3> Customizing the <i> toUpperCase() method of the </i> string prototype property </h3> <div id = "output"> </div> <script> let output = document.getElementById("output"); // Customizing the toUpperCase() method of the string prototype property to capitalize only the first character String.prototype.toUpperCase = function () { if (this.charCodeAt(0) >= 97 && this.charCodeAt(0) <= 122) { // convert to uppercase let ascii = this.charCodeAt(0) - 32; return String.fromCharCode(ascii) + this.slice(1); } else { return this; } } let str = "first letter of this string should be capitalized"; output.innerHTML = "Original string: " + str + "<br>" + "Capitalized string: " + str.toUpperCase(); </script> </body> </html>
Users learned to use the string prototype property. We can use it to add methods and properties to the string object. Also, we can use it to customize the string properties and methods. After adding methods into the string prototype, we can call the method by taking the string as a reference.