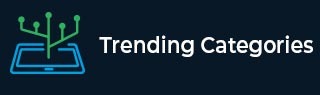
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
JavaScript regex - How to replace special characters?
Characters that are neither alphabetical nor numeric are known as special characters. Special characters are essentially all unreadable characters, including punctuation, accent, and symbol marks.
Remove any special characters from the string to make it easier to read and understand. Before we jump into the article let’s have a quick view on the regex expression in JavaScript.
Regex expression in JavaScript
Regular expressions are patterns that can be used to match character combinations in strings. Regular expressions are objects in JavaScript. These patterns can be used with the exec() and test() methods of RegExp as well as the match(), matchAll(), replace(), replaceAll(), search(), and split() methods of String.
Syntax
Following is the syntax for regex expression -
/pattern/modifier(s);
Let’s dive into the article for getting better understanding on how to replace special characters. For this we use replace() method.
Replace special characters using replace() method
The JavaScript built-in method string.replace() can be used to replace a portion of a supplied string with another string or a regular expression. The original string won't alter at all.
Syntax
Following is the syntax for replace()
string.replace(searchValue, newValue)
To learn more about replacing special characters, let’s look at the following examples.
Example 1
In the following example, we are running the script to replace all the special characters using replace().
<!DOCTYPE html> <html> <body style="background-color:#ABEBC6"> <script> var statement = "We#@lcome!! To! The@ Tutorials$Point%"; var result = statement.replace(/[^a-zA-Z ]/g, ""); document.write(result); </script> </body> </html>
When the script gets executed, it will generate an output consisting of text that has been replaced with special characters used in the script.
Example 2
Considering the following example, where we are running the script to replace special characters using replace().
<!DOCTYPE html> <html> <body> <p id="tutorial"></p> <button onclick="replacespecial()">Click To Change</button> <p id="tutorial1"></p> <script> var element_up = document.getElementById("tutorial"); var element_down = document.getElementById("tutorial1"); var statement = "Th@e B$es^t E-w#ay Le*arn(ing"; element_up.innerHTML = statement; function replacespecial() { element_down.innerHTML = statement.replace(/[&\/\#, +()$~%.'":@^*?<>{}]/g, '!'); } </script> </body> </html>
On running the above script, the output will pop up, displaying the original statement along with a click button. When the user clicks on the button, the event gets triggered and all the special characters are replaced with "!" displayed on the webpage.
Example 3
Execute the below script and observe how all the special characters are replaced using the replace()method.
<!DOCTYPE html> <html> <body> <script> let statement = "T!P, Wher$%e Y@ou F*ind L@ot O!f Courses" function replacespecial(_value){ var lowerCase = _value.toLowerCase(); var upperCase = _value.toUpperCase(); var replacement = ""; for(var i=0; i<lowerCase.length; ++i) { if(lowerCase[i] != upperCase[i] || lowerCase[i].trim() === '' || lowerCase[i].trim() === "." || lowerCase[i].trim() === ",") replacement += _value[i]; } return replacement; } let result = replacespecial(statement) document.write(result) </script> </body> </html>
When the script is executed, the event is triggered, and the text that was replaced from the special characters is displayed on the webpage.