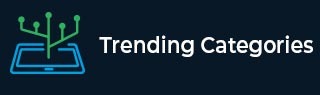
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
JavaScript program to check whether a given number is power of 2
The given number is a power of 2 if the number is generated using multiplying 2’s only. In this tutorial, we will learn to check whether a given number is a power of 2. Here, we will look at 5 different approaches to check whether a given number is a power of 2.
Using the Math.pow() Method
In JavaScript, the number can contain 64 bits most. So, we can use the for loop and Math.pow() method to find the 1 to 64 power of the 2. For loop, we can compare the ith power of 2 with the number. If it matches the number, we return true; Otherwise, we return false when the loop terminates.
Syntax
Users can follow the syntax below to use the for loop and Math.pow() method to check number is divisible by 2.
for () { if (Math.pow(2, i) == num) { return true; } }
Algorithm
Step 1 − Use for-loop and make iteration for i=1 to i=64 numbers.
Step 2 − In the for loop, use the Math.pow() method to get the ith power of 2.
Step 3 − Compare the ith power of the 2 with the number. If it matches, return true.
Step 4 − Return false if for loop terminates without returning true.
Example 1
In the example below, we have used the above approach to check whether a given number is a power of 2. In the output, users can observe that the checkPowOf2() function returns true or false based on the number is the power of 2.
<html> <body> <h3> Using the <i> Math.pow() method </i> to check whether the given number is a power of 2 or not </h3> <div id="output"> </div> <script> let output = document.getElementById('output'); let num1 = 342434535; let num2 = 2048; function checkPowOf2(num) { for (let i = 0; i < 64; i++) { if (Math.pow(2, i) == num) { return true; } } return false; } output.innerHTML += "The " + num1 + " is a power of 2 :- " + checkPowOf2(num1) + " <br> "; output.innerHTML += "The " + num2 + " is a power of 2 :- " + checkPowOf2(num2) + " <br> "; </script> </body> </html>
Using the Math.log() Method
We can take a logarithm of the number on base 2. If the number is an integer, it is a power of 2.
Syntax
Users can follow the syntax below to use the Math.log() method to check if the number is a power of 2.
let log = Math.log(number) / Math.log(2); let isInteger = parseInt(log) == log;
Example 2
In the example below, first, we take a logarithm of the number on the base of 2. After that, we use the parseInt() method to extract the integer from the logarithmic value, and if it is the same as the logarithmic value, the function returns true.
<html> <body> <h3> Using the <i> Math.log() method </i> to check whether the given number is a power of 2 or not </h3> <div id="output"> </div> <script> let output = document.getElementById('output'); let number1 = 1024; let number2 = 3454; function checkPowOf2(number) { // get a log of the number on base 2 let log = Math.log(number) / Math.log(2); // If the log is an integer, return true. if (parseInt(log) == log) { return true; } return false; } output.innerHTML += "The " + number1 + " is a power of 2 :- " + checkPowOf2(number1) + " <br> "; output.innerHTML += "The " + number2 + " is a power of 2 :- " + checkPowOf2(number2) + " <br> "; </script> </body> </html>
Determine by Counting the set Bits
If the number is a power of 2, it contains only a set bit. So, we can check every bit of the number one by one. If we get the first set bit, we set isSetBit equal to true. After that, if we get set bit again, we can say that number is not the power of 2.
Syntax
Users can follow the syntax below to determine whether the number is a power of 2 by counting the set bits.
while (number) { if (isSetBit) { return false; } else if (number & 1 == 1) { isSetBit = true; } number = number >> 1; }
Algorithm
Step 1 − Make iteration using a while loop while the number is not equal to zero.
Step 2 − Check if the value of the ‘isSetBit’ variable is true; return false.
Step 3 − If the value of the isSetBit variable is false, and the current bit is a set bit, change the value of the isSetBit variable to true.
Step 4 − Right shift the number by 1.
Example 3
In the example below, we iterate through the number using a while loop and check every bit of the number. We return false if we get the second set bit in the number.
<html> <body> <h3> Counting the <i> set bits </i> to check whether the given number is a power of 2 or not </h3> <div id="output"> </div> <script> let output = document.getElementById('output'); let number1 = 2048 * 2 * 2 * 2; let number2 = 87907; function checkPowOf2(number) { let isSetBit = false; if (number) { while (number) { if (isSetBit) { return false; } else if (number & 1 == 1) { isSetBit = true; } number = number >> 1; } return true; } return false; } output.innerHTML += "The " + number1 + " is a power of 2 :- " + checkPowOf2(number1) + " <br> "; output.innerHTML += "The " + number2 + " is a power of 2 :- " + checkPowOf2(number2) + " <br> "; </script> </body> </html>
Use the ‘&’ Operator
If the number is a power of 2, it contains only 1 in the leftmost bit. If we subtract 1 from the power of 2, the number contains 0 in the leftmost bit and 1 in other bits. So, if we do the ‘&’ operation between n and n-1, it always returns zero for all numbers equal to the power of 2.
Syntax
Users can follow the syntax below to use the ‘&’ operator to check given number is a power of 2.
let isPowerOf2 = number && !(number & number - 1)
Example 4
In the example below, first, we check if the number is not zero in the if statement. After that, we check if ‘n & n-1’ equals zero or not to decide whether the number is of power of 2.
<html> <body> <h3> Using the <i> & operator </i> to check whether the given number is a power of 2 or not </h3> <div id="output"> </div> <script> let output = document.getElementById('output'); let number1 = 1024 * 2 * 2 * 2; let number2 = 409540; function checkPowOf2(number) { if (number && !(number & number - 1)) { return true; } return false; } output.innerHTML += "The " + number1 + " is a power of 2 :- " + checkPowOf2(number1) + " <br> "; output.innerHTML += "The " + number2 + " is a power of 2 :- " + checkPowOf2(number2) + " <br> "; </script> </body> </html>