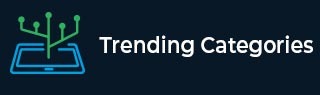
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
JavaScript Check whether string1 ends with strings2 or not
We are required to write a JavaScript function that takes in two strings, say, string1 and string2 and determines whether the string1 ends with string2 or not.
For example −
"The game is on" Here, "on" should return true
While,
"the game is off" Above, “of" should return false
Let's write the code for this function −
Example
const first = 'The game is on'; const second = ' on'; const endsWith = (first, second) => { const { length } = second; const { length: l } = first; const sub = first.substr(l - length, length); return sub === second; }; console.log(endsWith(first, second)); console.log(endsWith(first, ' off'));
Output
The output in the console will be −
true false
Advertisements