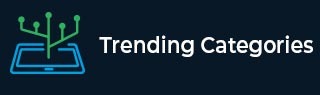
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
JavaScript algorithm for converting integers to roman numbers
Let’s say, we are required to write a function, say intToRoman(), which, as the name suggests, returns a Roman equivalent of the number passed in it as an argument.
Let’s write the code for this function −
Example
const intToRoman = (num) => { let result = ""; while(num){ if(num>=1000){ result += "M"; num -= 1000; }else if(num>=500){ if(num>=900){ result += "CM"; num -= 900; }else{ result += "D"; num -= 500; } }else if(num>=100){ if(num>=400){ result += "CD"; num -= 400; }else{ result += "C"; num -= 100; } }else if(num>=50){ if(num>=90){ result += "XC"; num -= 90; }else{ result += "L"; num -= 50; } }else if(num>=10){ if(num>=40){ result += "XL"; num -= 40; }else{ result += "X"; num -= 10; } }else if(num>=5){ if(num>=9){ result += "IX"; num -= 9; }else{ result += "V"; num -= 5; } }else{ if(num>=4){ result += "IV"; num -= 4; }else{ result += "I"; num -= 1; } } } return result; }; console.log(intToRoman(178)); console.log(intToRoman(89)); console.log(intToRoman(55)); console.log(intToRoman(1555));
Output
The output for this code in the console will be −
CLXXVIII LXXXIX LV MDLV
Advertisements