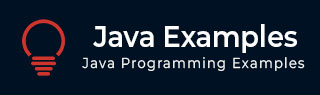
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to remove the white spaces in Java
Problem Description
How to remove the white spaces?
Solution
Following example demonstrates how to remove the white spaces with the help matcher.replaceAll(stringname) method of Util.regex.Pattern class.
import java.util.regex.Matcher; import java.util.regex.Pattern; public class Main { public static void main(String[] argv) { String str = "This is a Java program. This is another Java Program."; String pattern="[\\s]"; String replace = ""; Pattern p = Pattern.compile(pattern); Matcher m = p.matcher(str); str = m.replaceAll(replace); System.out.println(str); } }
Result
The above code sample will produce the following result.
ThisisaJavaprogram.ThisisanotherJavaprogram.
The following is an example to remove the white spaces.
import java.util.Scanner ; import java.lang.String ; public class Main { public static void main (String[]args) { String s1 = null; Scanner scan = new Scanner(System.in); System.out.println("Enter your string\n"); s1 = scan.nextLine(); System.out.println("Input String is :\n"+s1); String s2 = s1.replaceAll("\\s+",""); System.out.println("\n Output String is :\n"+s2); } }
The above code sample will produce the following result.
Enter your string sairamkrishna mammahe Input String is : sairamkrishna mammahe Output String is : sairamkrishnamammahe
java_regular_exp.htm
Advertisements