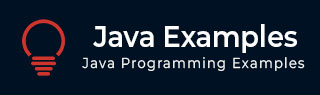
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to find hostname from IP Address in Java
Problem Description
How to find hostname from IP Address?
Solution
Following example shows how to change the host name to its specific IP address with the help of InetAddress.getByName() method of net.InetAddress class.
import java.net.InetAddress; public class Main { public static void main(String[] argv) throws Exception { InetAddress addr = InetAddress.getByName("74.125.67.100"); System.out.println("Host name is: "+addr.getHostName()); System.out.println("Ip address is: "+ addr.getHostAddress()); } }
Result
The above code sample will produce the following result.
Host name is: 100.67.125.74.bc.googleusercontent.com Ip address is: 74.125.67.100
The following is an example to find hostname from IP Address
import java.net.InetAddress; import java.net.UnknownHostException; public class NewClass1 { public static void main(String[] args) { InetAddress ip; String hostname; try { ip = InetAddress.getLocalHost(); hostname = ip.getHostName(); System.out.println("Your current IP address : " + ip); System.out.println("Your current Hostname : " + hostname); } catch (UnknownHostException e) { e.printStackTrace(); } } }
The above code sample will produce the following result.
Your current IP address : localhost/127.0.0.1 Your current Hostname : localhost
java_networking.htm
Advertisements