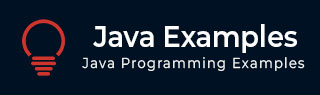
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to split a string into a number of substrings in Java
Problem Description
How to split a string into a number of substrings?
Solution
Following example shows how to change the host name to its specific IP address with the help of InetAddress.getByName() method of net.InetAddress class.
import java.net.InetAddress; import java.net.UnknownHostException; public class GetIP { public static void main(String[] args) { InetAddress address = null; try { address = InetAddress.getByName("www.javatutorial.com"); } catch (UnknownHostException e) { System.exit(2); } System.out.println(address.getHostName() + "=" + address.getHostAddress()); System.exit(0); } }
Result
The above code sample will produce the following result.
http://www.javatutorial.com = 123.14.2.35
The following is an another example of getHostAddress() and getHostName() in Java
import java.net.InetAddress; import java.net.UnknownHostException; public class Demo { public static void main(String[] args) { InetAddress ipadd; String hostname; try { ipadd = InetAddress.getLocalHost(); hostname = ipadd.getHostName(); System.out.println("Your IP address : " + ipadd); System.out.println("Your Hostname : " + hostname); } catch (UnknownHostException e) { } } }
The above code sample will produce the following result.
Your IP address : 4d623edc62d4/172.17.0.2 Your Hostname : 4d623edc62d4
java_networking.htm
Advertisements