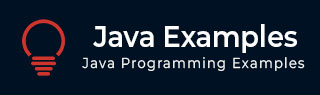
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to draw text using GUI in Java
Problem Description
How to draw text using GUI?
Solution
Following example demonstrates how to draw text drawString(), setFont() methods of Graphics class.
import java.awt.Font; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.RenderingHints; import javax.swing.JFrame; import javax.swing.JPanel; public class Main extends JPanel{ public void paint(Graphics g) { Graphics2D g2 = (Graphics2D)g; g2.setRenderingHint( RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); Font font = new Font("Serif", Font.PLAIN, 96); g2.setFont(font); g2.drawString("Text", 40, 120); } public static void main(String[] args) { JFrame f = new JFrame(); f.getContentPane().add(new Main()); f.setSize(300, 200); f.setVisible(true); } }
Result
The above code sample will produce the following result.
Text is displayed in a frame.
The following is an example to draw text using GUI.
import java.awt.Font; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.RenderingHints; import javax.swing.JFrame; import javax.swing.JPanel; public class Panel extends JPanel { public void paint(Graphics gr) { Graphics2D g = (Graphics2D)gr; g.setRenderingHint( RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); Font font = new Font("Serif", Font.PLAIN, 96); g.setFont(font); g.drawString("Tutorialspoint", 40, 120); } public static void main(String[] args) { JFrame f = new JFrame(); f.getContentPane().add(new Panel()); f.setSize(300, 200); f.setVisible(true); } }
Result
The above code sample will produce the following result.
Text is displayed in a frame.
java_simple_gui.htm
Advertisements