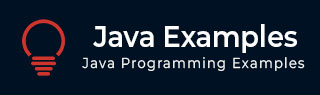
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to draw a solid rectangle using GUI in Java
Problem Description
How to draw a solid rectangle using GUI?
Solution
Following example demonstrates how to display a solid rectangle using fillRect() method of Graphics class.
import java.awt.Graphics; import javax.swing.JFrame; import javax.swing.JPanel; public class Main extends JPanel { public static void main(String[] a) { JFrame f = new JFrame(); f.setSize(400, 400); f.add(new Main()); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.setVisible(true); } public void paint(Graphics g) { g.fillRect (5, 15, 50, 75); } }
Result
The above code sample will produce the following result.
Solid rectangle is created.
The following is an example to draw a solid rectangle using GUI.
import java.awt.Color; import java.awt.Graphics; import java.awt.Graphics2D; import javax.swing.JFrame; import javax.swing.JPanel; public class Panel extends JPanel { public void paintComponent(Graphics g) { super.paintComponent(g); Graphics2D g2d = (Graphics2D) g; g2d.setColor(new Color(31, 21, 1)); g2d.fillRect(250, 195, 90, 60); } public static void main(String[] args) { Panel rects = new Panel(); JFrame frame = new JFrame("Rectangles"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(rects); frame.setSize(360, 300); frame.setLocationRelativeTo(null); frame.setVisible(true); } }
The above code sample will produce the following result.
Solid rectangle is created.
java_simple_gui.htm
Advertisements