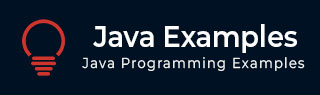
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to format the text in a word document using Java
Problem Description
How to format the text in a word document using Java.
Solution
Following is the program to format the text in a word document using Java.
import java.io.File; import java.io.FileOutputStream; import org.apache.poi.xwpf.usermodel.VerticalAlign; import org.apache.poi.xwpf.usermodel.XWPFDocument; import org.apache.poi.xwpf.usermodel.XWPFParagraph; import org.apache.poi.xwpf.usermodel.XWPFRun; public class FormatTextInWord { public static void main(String[] args)throws Exception { //Blank Document XWPFDocument document = new XWPFDocument(); //Write the Document in file system FileOutputStream out = new FileOutputStream(new File("C:/poiword/fontstyle.docx")); //create paragraph XWPFParagraph paragraph = document.createParagraph(); //Set Bold an Italic XWPFRun paragraphOneRunOne = paragraph.createRun(); paragraphOneRunOne.setBold(true); paragraphOneRunOne.setItalic(true); paragraphOneRunOne.setText("Font Style"); paragraphOneRunOne.addBreak(); //Set text Position XWPFRun paragraphOneRunTwo = paragraph.createRun(); paragraphOneRunTwo.setText("Font Style two"); paragraphOneRunTwo.setTextPosition(100); //Set Strike through and Font Size and Subscript XWPFRun paragraphOneRunThree = paragraph.createRun(); paragraphOneRunThree.setStrike(true); paragraphOneRunThree.setFontSize(20); paragraphOneRunThree.setSubscript(VerticalAlign.SUBSCRIPT); paragraphOneRunThree.setText(" Different Font Styles"); document.write(out); out.close(); System.out.println("fontstyle.docx written successully"); } }
Output
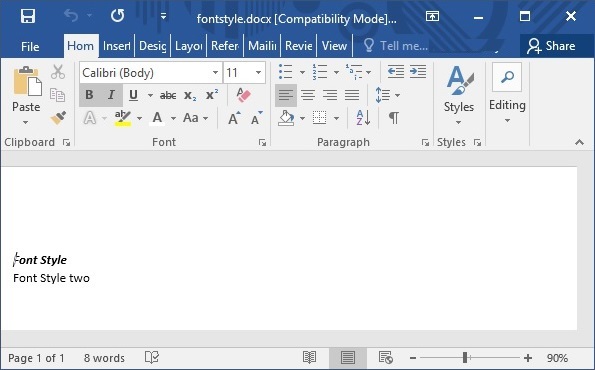
java_apache_poi_word
Advertisements