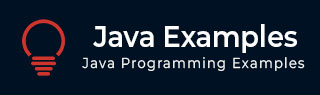
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to get a files size in bytes using Java
Problem Description
How to get a files size in bytes?
Solution
This example shows how to get a file's size in bytes by using file.exists() and file.length() method of File class.
import java.io.File; public class Main { public static long getFileSize(String filename) { File file = new File(filename); if (!file.exists() || !file.isFile()) { System.out.println("File doesn\'t exist"); return -1; } return file.length(); } public static void main(String[] args) { long size = getFileSize("c:/java.txt"); System.out.println("Filesize in bytes: " + size); } }
Result
The above code sample will produce the following result.To test this example, first create a text file 'java.txt' in 'C' drive.The size may vary depending upon the size of the file.
File size in bytes: 480
The following is another sample example of files size in java
import java.io.File; public class FileSizeExample { public static void main(String[] args) { File file = new File("C:\\Users\\TutorialsPoint7\\Desktop\\abc.png"); if(file.exists()) { double bytes = file.length(); double kilobytes = (bytes / 1024); double megabytes = (kilobytes / 1024); double gigabytes = (megabytes / 1024); double terabytes = (gigabytes / 1024); double petabytes = (terabytes / 1024); double exabytes = (petabytes / 1024); double zettabytes = (exabytes / 1024); double yottabytes = (zettabytes / 1024); System.out.println("bytes : " + bytes); System.out.println("kilobytes : " + kilobytes); System.out.println("megabytes : " + megabytes); System.out.println("gigabytes : " + gigabytes); System.out.println("terabytes : " + terabytes); System.out.println("petabytes : " + petabytes); System.out.println("exabytes : " + exabytes); System.out.println("zettabytes : " + zettabytes); System.out.println("yottabytes : " + yottabytes); } else { System.out.println("File does not exists!"); } } }
The above code sample will produce the following result.To test this example, first create a text file 'java.txt' in 'C' drive.The size may vary depending upon the size of the file.
bytes : 6119.0 kilobytes : 5.9755859375 megabytes : 0.005835533142089844 gigabytes : 5.698762834072113E-6 terabytes : 5.565198080148548E-9 petabytes : 5.434763750145066E-12 exabytes : 5.307386474751041E-15 zettabytes : 5.182994604249064E-18 yottabytes : 5.061518168211976E-21
java_files.htm
Advertisements