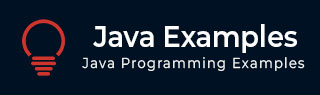
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to rename a file in Java
Problem Description
How to rename a file?
Solution
This example demonstrates how to renaming a file using oldName.renameTo(newName) method of File class.
import java.io.File; public class Main { public static void main(String[] args) { File oldName = new File("C:/program.txt"); File newName = new File("C:/java.txt"); if(oldName.renameTo(newName)) { System.out.println("renamed"); } else { System.out.println("Error"); } } }
Result
The above code sample will produce the following result.To test this example, first create a file 'program.txt' in 'C' drive.
renamed
The following is another example of rename a file in java
import java.io.File; public class RenameFileExample { public static void main(String[] args) { File oldfile = new File("C:/oldfile_name.txt"); File newfile = new File("C:/newfile_name.txt"); if(oldfile.renameTo(newfile)) { System.out.println("File name changed succesful"); } else { System.out.println("Rename failed"); } } }
Result
The above code sample will produce the following result. To test this example, first create a file 'oldfile_name.txt' in 'C' drive.
File name changed succesful
java_files.htm
Advertisements