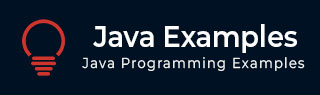
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to check a file exist or not in Java
Problem Description
How to check a file exist or not?
Solution
This example shows how to check a files existence by using file.exists() method of File class.
import java.io.File; public class Main { public static void main(String[] args) { File file = new File("C:/java.txt"); System.out.println(file.exists()); } }
Result
The above code sample will produce the following result (if the file "java.txt" exists in 'C' drive).
false
The following is an another sample example of file exist or not in java
import java.io.File; import java.io.FileNotFoundException; import java.io.IOException; import java.io.PrintpWriter; import java.nio.file.FileAlreadyExistsException; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; public class fileexist { public static void main(String[] args) throws IOException { File f = new File(System.getProperty("user.dir")+"/folder/file.txt"); System.out.println(f.exists()); if(!f.getParentFile().exists()){ f.getParentFile().mkdirs(); } if(!f.exists()){ try { f.createNewFile(); } catch (Exception e) { e.printStackTrace(); } } try { File dir = new File(f.getParentFile(), f.getName()); PrintpWriter pWriter = new PrintpWriter(dir); pWriter.print("writing anything..."); pWriter.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } } }
The above code sample will produce the following result (if the file "java.txt" exists in 'C' drive).
true
java_files.htm
Advertisements