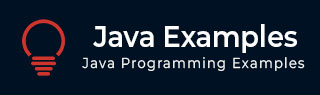
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to create a temporary file in Java
Problem Description
How to create a temporary file?
Solution
This example shows how to create a temporary file using createTempFile() method of File class.
import java.io.*; public class Main { public static void main(String[] args) throws Exception { File temp = File.createTempFile ("pattern", ".suffix"); temp.deleteOnExit(); BufferedWriter out = new BufferedWriter (new FileWriter(temp)); out.write("aString"); System.out.println("temporary file created:"); out.close(); } }
Result
The above code sample will produce the following result.
temporary file created:
The following is another sample example of create a temporary file in java
import java.io.File; import java.io.IOException; public class CreateTempFileExample { public static void main(String[] args) { try { File f1 = File.createTempFile("temp-file-name", ".tmp"); System.out.println("Temp file : " + f1.getAbsolutePath()); } catch(IOException e) { e.printStackTrace(); } } }
The above code sample will produce the following result.
Temp file : C:\Users\TUTORI~1\AppData\Local\Temp\temp-file-name3671918809488333932.tmp
java_files.htm
Advertisements