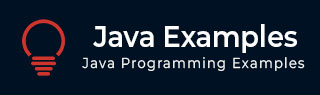
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to get the size of a directory in Java
Problem Description
How to get the size of a directory?
Solution
Following example shows how to get the size of a directory with the help of FileUtils.sizeofDirectory(File Name) method of FileUtils class.
import java.io.File; import org.apache.commons.io.FileUtils; public class Main { public static void main(String[] args) { long size = FileUtils.sizeOfDirectory(new File("C:/Windows")); System.out.println("Size: " + size + " bytes"); } }
Result
The above code sample will produce the following result.
Size: 2048 bytes
The following is an another sample example to get the size of a directory in Java
import java.io.File; import java.util.ArrayList; public class Main { public static void main(String[] args) { long folderSize = findSize("C:\\a"); System.out.println("Size in byte :"+folderSize); } public static long findSize(String path) { long totalSize = 0; ArrayList<String> directory = new ArrayList<String>(); File file = new File(path); if(file.isDirectory()) { directory.add(file.getAbsolutePath()); while (directory.size() > 0) { String folderPath = directory.get(0); System.out.println("Size of this :"+folderPath); directory.remove(0); File folder = new File(folderPath); File[] filesInFolder = folder.listFiles(); int noOfFiles = filesInFolder.length; for(int i = 0 ; i < noOfFiles ; i++) { File f = filesInFolder[i]; if(f.isDirectory()) { directory.add(f.getAbsolutePath()); } else { totalSize += f.length(); } } } } else { totalSize = file.length(); } return totalSize; } }
The above code sample will produce the following result.
Size of this :C:\a Size of this :C:\a\b Size of this :C:\a\b\c Size of this :C:\a\b\c\d Size in byte :0
java_directories.htm
Advertisements