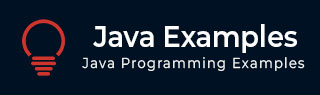
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to display name of the weekdays in Java
Problem Description
How to display name of the weekdays?
Solution
This example displays the names of the weekdays in short form with the help of DateFormatSymbols().getWeekdays() method of DateFormatSymbols class.
import java.text.SimpleDateFormat; import java.text.DateFormatSymbols; public class Main { public static void main(String[] args) { String[] weekdays = new DateFormatSymbols().getWeekdays(); for (int i = 2; i < (weekdays.length-1); i++) { String weekday = weekdays[i]; System.out.println("weekday = " + weekday); } } }
Result
The above code sample will produce the following result.
weekday = Monday weekday = Tuesday weekday = Wednesday weekday = Thursday weekday = Friday
The following is an another sample example of display name of the weekdays
import java.text.Format; import java.text.SimpleDateFormat; import java.util.Date; import java.util.Locale; import java.util.Calendar; public class Main { public static void main(String[] argv) throws Exception { Date d = new Date(); SimpleDateFormat dateFormat = new SimpleDateFormat("EEE", Locale.US); String str = dateFormat.format(d); System.out.println(str); } }
The above code sample will produce the following result.
Fri
java_date_time.htm
Advertisements