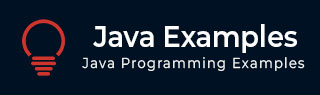
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to execute binary search on a vector using Java
Problem Description
How to execute binary search on a vector?
Solution
Following example how to execute binary search on a vector with the help of v.add() method of Vector class and sort.Collection() method of Collection class.
import java.util.Collections; import java.util.Vector; public class Main { public static void main(String[] args) { Vector<String> v = new Vector<String>(); v.add("X"); v.add("M"); v.add("D"); v.add("A"); v.add("O"); Collections.sort(v); System.out.println(v); int index = Collections.binarySearch(v, "D"); System.out.println("Element found at : " + index); } }
Result
The above code sample will produce the following result.
[A, D, M, O, X] Element found at : 1
The following is an another example to execute binary search on a vector with the help of v.add() method of Vector class and sort.Collection() method of Collection class.
import java.util.Vector; import java.util.Collections; public class Demo { public static void main(String[] args) { Vector vec = new Vector(); vec.add("X"); vec.add("M"); vec.add("D"); vec.add("A"); vec.add("O"); Collections.sort(vec); System.out.println("Sorted Vector: " + vec); int found = Collections.binarySearch(vec, "D"); System.out.println("Element found at : " + found); } }
The above code sample will produce the following result.
Sorted Vector: [A, D, M, O, X] Element found at : 1
java_data_structure.htm
Advertisements