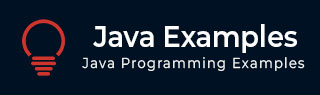
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to get the first and the last element of a linked list in Java
Problem Description
How to get the first and the last element of a linked list?
Solution
Following example shows how to get the first and last element of a linked list with the help of linkedlistname.getFirst() and linkedlistname.getLast() of LinkedList class.
import java.util.LinkedList; public class Main { public static void main(String[] args) { LinkedList<String> lList = new LinkedList<String>(); lList.add("100"); lList.add("200"); lList.add("300"); lList.add("400"); lList.add("500"); System.out.println("First element of LinkedList is : " + lList.getFirst()); System.out.println("Last element of LinkedList is : " + lList.getLast()); } }
Result
The above code sample will produce the following result.
First element of LinkedList is :100 Last element of LinkedList is :500
The following is an another example to get the first and the last element of a linked list
import java.util.LinkedList; public class Demo { public static void main(String[] args) { LinkedList lList = new LinkedList(); lList.add("1"); lList.add("2"); lList.add("3"); lList.add("4"); lList.add("5"); System.out.println("First element is : " + lList.getFirst()); System.out.println("Last element is : " + lList.getLast()); } }
The above code sample will produce the following result.
First element is : 1 Last element is : 5
java_data_structure.htm
Advertisements