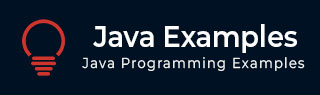
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to compare elements in a collection using Java
Problem Description
How to compare elements in a collection?
Solution
Following example compares the element of a collection by converting a string into a treeset using Collection.min() and Collection.max() methods of Collection class.
import java.util.Collections; import java.util.Set; import java.util.TreeSet; public class MainClass { public static void main(String[] args) { String[] coins = { "Penny", "nickel", "dime", "Quarter", "dollar" }; Set set = new TreeSet(); for (int i = 0; i < coins.length; i++)set.add(coins[i]); System.out.println(Collections.min(set)); System.out.println(Collections.min(set, String.CASE_INSENSITIVE_ORDER)); for(int i = 0; i <= 10; i++)System.out.print('-'); System.out.println(Collections.max(set)); System.out.println(Collections.max(set, String.CASE_INSENSITIVE_ORDER)); } }
Result
The above code sample will produce the following result.
Penny dime ---------- nickle Quarter
java_collections.htm
Advertisements