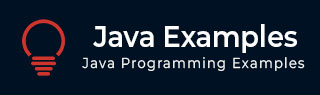
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to find common elements from arrays in Java
Problem Description
How to find common elements from arrays?
Solution
Following example shows how to find common elements from two arrays and store them in an array.
import java.util.ArrayList; public class NewClass { public static void main(String[] args) { ArrayList objArray = new ArrayList(); ArrayList objArray2 = new ArrayList(); objArray2.add(0,"common1"); objArray2.add(1,"common2"); objArray2.add(2,"notcommon"); objArray2.add(3,"notcommon1"); objArray.add(0,"common1"); objArray.add(1,"common2"); objArray.add(2,"notcommon2"); System.out.println("Array elements of array1"+objArray); System.out.println("Array elements of array2"+objArray2); objArray.retainAll(objArray2); System.out.println("Array1 after retaining common elements of array2 & array1"+objArray); } }
Result
The above code sample will produce the following result.
Array elements of array1[common1, common2, notcommon2] Array elements of array2[common1, common2, notcommon, notcommon1] Array1 after retaining common elements of array2 & array1 [common1, common2]
Another sample example of Find common elements from arrays
public class HelloWorld { public static void main(String a[]) { int[] arr1 = {4,7,3,9,2}; int[] arr2 = {3,2,12,9,40,32,4}; for(int i = 0;i < arr1.length; i++) { for(int j = 0; j < arr2.length; j++) { if(arr1[i] == arr2[j]) { System.out.println(arr1[i]); } } } } }
The above code sample will produce the following result.
4 3 9 2
java_arrays.htm
Advertisements