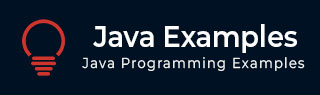
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to extend an array after initialisation in Java
Problem Description
How to extend an array after initialisation?
Solution
Following example shows how to extend an array after initialization by creating an new array.
public class Main { public static void main(String[] args) { String[] names = new String[] { "A", "B", "C" }; String[] extended = new String[5]; extended[3] = "D"; extended[4] = "E"; System.arraycopy(names, 0, extended, 0, names.length); for (String str : extended){ System.out.println(str); } } }
Result
The above code sample will produce the following result.
A B C D E
The following is an another Sample example of arrays expansion
public class Main { public void extendArraySize() { String[] names = new String[] {"Sai", "Ram", "Krishna"}; String[] extended = new String[5]; extended[3] = "Prasad"; extended[4] = "Mammahe"; System.arraycopy(names, 0, extended, 0, names.length); for (String str : extended) System.out.println(str); } public static void main(String[] args) { new Main().extendArraySize(); } }
The above code sample will produce the following result.
Sai Ram Krishna Prasad Mammahe
java_arrays.htm
Advertisements