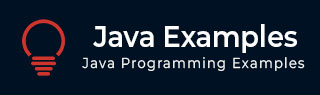
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to add water marks to the images in a PDF using Java
Problem Description
How to add water marks to the images in a PDF using Java.
Solution
Following is the program to add water marks to the images in a PDF using Java.
import com.itextpdf.io.image.ImageDataFactory; import com.itextpdf.kernel.color.DeviceGray; import com.itextpdf.kernel.geom.Rectangle; import com.itextpdf.kernel.pdf.PdfDocument; import com.itextpdf.kernel.pdf.PdfWriter; import com.itextpdf.kernel.pdf.xobject.PdfFormXObject; import com.itextpdf.layout.Canvas; import com.itextpdf.layout.Document; import com.itextpdf.layout.element.Image; import com.itextpdf.layout.property.TextAlignment; public class AddingToImagesInPDF { public static void main(String args[]) throws Exception { String file = "C:/EXAMPLES/itextExamples/addingImageToPDF.pdf"; //Creating a PdfDocument object PdfDocument pdfDoc = new PdfDocument(new PdfWriter(file)); //Creating a Document object Document doc = new Document(pdfDoc); //Creating an Image Image image = new Image(ImageDataFactory.create( "C:/EXAMPLES/itextExamples/logo.jpg")); image.scaleToFit(400, 700); //Creating template PdfFormXObject template = new PdfFormXObject(new Rectangle( image.getImageScaledWidth(), image.getImageScaledHeight())); Canvas canvas = new Canvas(template, pdfDoc).add(image); String watermark = "Welcome to Tutorialspoint"; canvas.setFontColor(DeviceGray.LIGHT_GRAY) .showTextAligned(watermark, 100, 360, TextAlignment.CENTER); //Adding template to document Image image1 = new Image(template); doc.add(image1); //Closing the document doc.close(); System.out.println("Watermark added successfully.."); } }
Input
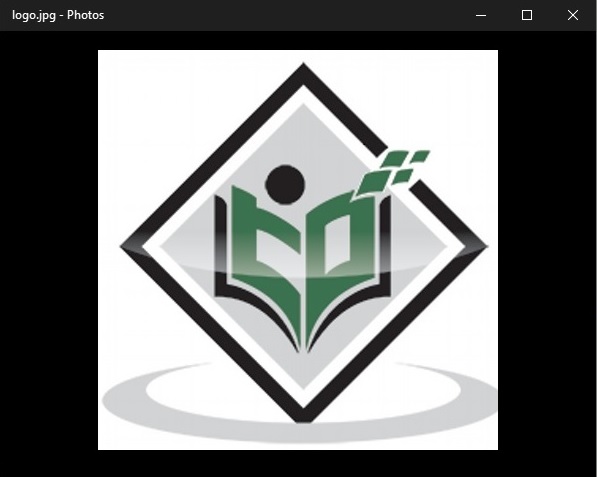
Output
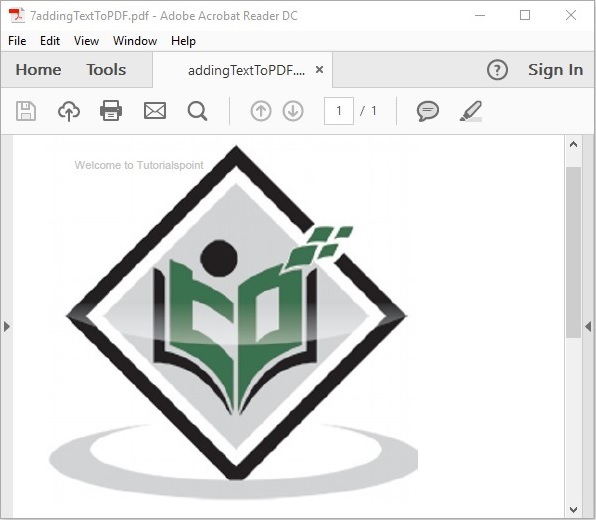
java_itext
Advertisements