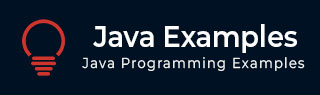
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to add text to a PDF document as paragraphs using Java
Problem Description
How to add text to a PDF document as paragraphs using Java.
Solution
Following is the program to add text to a PDF document as paragraphs using Java.
import com.itextpdf.io.font.FontConstants; import com.itextpdf.kernel.color.Color; import com.itextpdf.kernel.font.PdfFontFactory; import com.itextpdf.kernel.pdf.PdfDocument; import com.itextpdf.kernel.pdf.PdfWriter; import com.itextpdf.layout.Document; import com.itextpdf.layout.element.Paragraph; import com.itextpdf.layout.element.Text; public class AddingParagraphsToPdf { public static void main(String args[]) throws Exception { String file = "C:/EXAMPLES/itextExamples/formatingTextInPDF.pdf"; //Creating a PdfDocument object PdfDocument pdfDoc = new PdfDocument(new PdfWriter(file)); //Creating a Document object Document doc = new Document(pdfDoc); //Adding text to the document Text text1 = new Text("Tutorials Point originated from the idea that there exists a class of readers who respond better to online content and prefer to learn new skills at their own pace from the comforts of their drawing rooms."); //Setting color to the text text1.setFontColor(Color.RED); //Setting font to the text text1.setFont(PdfFontFactory.createFont(FontConstants.HELVETICA)); //Creating a paragraph 1 Paragraph para1 = new Paragraph(text1); Text text2 = new Text("The journey commenced with a single tutorial on HTML in 2006 and elated by the response it generated, we worked our way to adding fresh tutorials to our repository which now proudly flaunts a wealth of tutorials and allied articles on topics ranging from programming languages to web designing to academics and much more."); //Setting color to the text text2.setFontColor(Color.RED); //Setting font to the text text2.setFont(PdfFontFactory.createFont(FontConstants.HELVETICA)); //Creating a paragraph 2 Paragraph para2 = new Paragraph(text2); //Adding paragraphs to the document doc.add(para1); doc.add(para2); //Closing the document doc.close(); System.out.println("Text added successfully.."); } }
Output
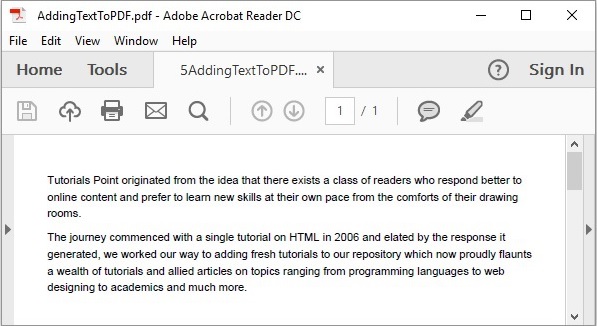
java_itext
Advertisements