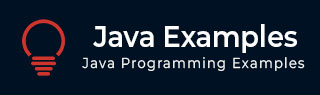
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to add images to a table using Java
Problem Description
How to add images to a table using Java.
Solution
Following is the program to add images to a table using Java.
import com.itextpdf.io.image.ImageDataFactory; import com.itextpdf.kernel.pdf.PdfDocument; import com.itextpdf.kernel.pdf.PdfWriter; import com.itextpdf.layout.Document; import com.itextpdf.layout.element.Cell; import com.itextpdf.layout.element.Image; import com.itextpdf.layout.element.Table; public class AddingImageToTable { public static void main(String args[]) throws Exception { String file = "C:/EXAMPLES/itextExamples/addingImageToTable.pdf"; //Creating a PdfDocument object PdfDocument pdfDoc = new PdfDocument(new PdfWriter(file)); //Creating a Document object Document doc = new Document(pdfDoc); //Creating a table Table table = new Table(2); //Adding cells to the table table.addCell(new Cell().add("Tutorial ID")); table.addCell(new Cell().add("1")); table.addCell(new Cell().add("Tutorial Title")); table.addCell(new Cell().add("JavaFX")); table.addCell(new Cell().add("Tutorial Author")); table.addCell(new Cell().add("Krishna Kasyap")); table.addCell(new Cell().add("Submission date")); table.addCell(new Cell().add("2016-07-06")); table.addCell(new Cell().add("Tutorial Icon")); //Adding image to the cell in a table Image img = new Image(ImageDataFactory.create( "C:/EXAMPLES/itextExamples/javafxLogo.jpg")); table.addCell(img.setAutoScale(true)); //Adding Table to document doc.add(table); //Closing the document doc.close(); System.out.println("Image added successfully.."); } }
Output
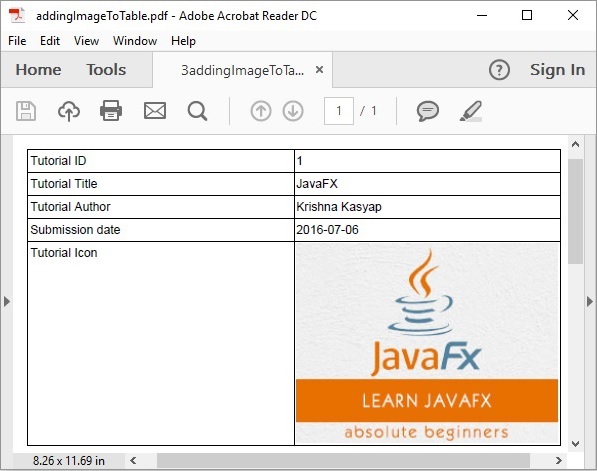
java_itext
Advertisements