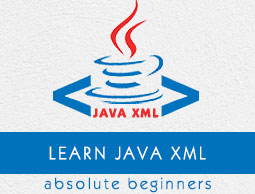
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
Javax.xml.bind.JAXBContext.createJAXBIntrospector() Method
Description
The Javax.xml.bind.JAXBContext.createJAXBIntrospector() method creates a JAXBIntrospector object that can be used to introspect JAXB objects.
Declaration
Following is the declaration for javax.xml.bind.JAXBContext.createJAXBIntrospector() method
public JAXBIntrospector createJAXBIntrospector()
Parameters
NA
Return Value
The method always return a non-null valid JAXBIntrospector object.
Exception
UnsupportedOperationException − Calling this method on JAXB 1.0 implementations will throw an UnsupportedOperationException.
Example
The following example shows the usage of javax.xml.bind.JAXBContext.createJAXBIntrospector() method. To proceed, consider the following Student class which will be used to have objects for marshalling purpose −
package com.tutorialspoint; import javax.xml.bind.annotation.XmlAttribute; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement public class Student{ String name; int age; int id; public String getName(){ return name; } @XmlElement public void setName(String name){ this.name = name; } public int getAge(){ return age; } @XmlElement public void setAge(int age){ this.age = age; } public int getId(){ return id; } @XmlAttribute public void setId(int id){ this.id = id; } }
The program below creates JAXB introspector which is used for introspect the JAXB object.
package com.tutorialspoint; import javax.xml.bind.JAXBContext; import javax.xml.bind.JAXBElement; import javax.xml.bind.JAXBIntrospector; import javax.xml.bind.Marshaller; import javax.xml.namespace.QName; public class JAXBContextDemo { public static void main(String[] args) { JAXBElement jElement = null; try { // object value is assigned to "Sania" Object value = "Sania"; // JAXB context object created JAXBContext jc = JAXBContext.newInstance(String.class); // JAXBIntrospector object is created JAXBIntrospector introspector = jc.createJAXBIntrospector(); // marshaller created Marshaller marshaller = jc.createMarshaller(); if(null == introspector.getElementName(value)) { QName qName = new QName("Name"); jElement = new JAXBElement(qName, Object.class, value); marshaller.marshal(jElement, System.out); } else { marshaller.marshal(value, System.out); } }catch(Exception ex) { ex.printStackTrace(); } } }
Before we proceed for compilation, we need to make sure that that we download JAXB2.xxx.jar and put it in our CLASSPATH. Once setup is ready, let us compile and run the above program, this will produce the following result −
<?xml version = "1.0" encoding = "UTF-8" standalone = "yes"?> <Name xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xmlns:xs = "http://www.w3.org/2001/XMLSchema" xsi:type = "xs:string">Sania</Name>