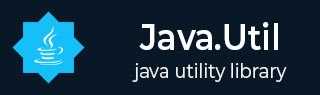
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java TimerTask scheduledExecutionTime() Method
Description
The scheduledExecutionTime() method is used to return the scheduled execution time of the most recent actual execution of this task.
Declaration
Following is the declaration for java.util.TimerTask.scheduledExecutionTime() method.
public long scheduledExecutionTime()
Parameters
NA
Return Value
The method call returns the time at which the most recent execution of this task was scheduled to occur.
Exception
NA
Running a Timer Scheduled At Fixed Rate Example
The following example shows the usage of Java Timer scheduledExecutionTime() method to print the time of execution of most recent execution of the task. We've created a timer object using a CustomTimerTask object. CustomTimerTask is custom class extending TimerTask class and implements the run() method which will execute at scheduled time. Then we created a timer object and scheduled a task using scheduleAtFixedRate() to execute at every 100 milliseconds starting from now. Then we've printed the time of execution using scheduledExecutionTime() method.
package com.tutorialspoint; import java.util.Date; import java.util.Timer; import java.util.TimerTask; public class TimerTaskDemo { public static void main(String[] args) { // creating timer task, timer TimerTask tasknew = new CustomTimerTask(); Timer timer = new Timer(); // scheduling the task timer.scheduleAtFixedRate(tasknew, new Date(), 100); // checking scheduled execution time System.out.println("Time is :"+tasknew.scheduledExecutionTime()); try { Thread.sleep(500); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } class CustomTimerTask extends TimerTask { @Override public void run() { System.out.println("working on"); } }
Output
Let us compile and run the above program, this will produce the following result.
Time is :1675410212458 working on working on working on working on working on working on
Running a Timer as Daemon Process Scheduled At Fixed Rate Example
The following example shows the usage of Java Timer scheduledExecutionTime() method to print the time of execution of most recent execution of the task. We've created a timer object using a CustomTimerTask object. CustomTimerTask is custom class extending TimerTask class and implements the run() method which will execute at scheduled time. Then we created a timer object as a daemon thread and scheduled a task using scheduleAtFixedRate() to execute at every 100 milliseconds starting from now. Then we've printed the time of execution using scheduledExecutionTime() method.
package com.tutorialspoint; import java.util.Date; import java.util.Timer; import java.util.TimerTask; public class TimerTaskDemo { public static void main(String[] args) { // creating timer task, timer TimerTask tasknew = new CustomTimerTask(); Timer timer = new Timer(true); // scheduling the task timer.scheduleAtFixedRate(tasknew, new Date(), 100); // checking scheduled execution time System.out.println("Time is :"+tasknew.scheduledExecutionTime()); try { Thread.sleep(500); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } class CustomTimerTask extends TimerTask { @Override public void run() { System.out.println("working on"); } }
Output
Let us compile and run the above program, this will produce the following result.
Time is :1675410564119 working on working on working on working on working on working on
Running a Named Timer as Daemon Process Scheduled At Fixed Rate Example
The following example shows the usage of Java Timer scheduledExecutionTime() method to print the time of execution of most recent execution of the task. We've created a timer object using a CustomTimerTask object. CustomTimerTask is custom class extending TimerTask class and implements the run() method which will execute at scheduled time. Then we created a timer object as a daemon thread, with a given name and scheduled a task using scheduleAtFixedRate() to execute at every 100 milliseconds starting from now. Then we've printed the time of execution using scheduledExecutionTime() method.
package com.tutorialspoint; import java.util.Date; import java.util.Timer; import java.util.TimerTask; public class TimerTaskDemo { public static void main(String[] args) { // creating timer task, timer TimerTask tasknew = new CustomTimerTask(); Timer timer = new Timer("test",true); // scheduling the task timer.scheduleAtFixedRate(tasknew, new Date(), 100); // checking scheduled execution time System.out.println("Time is :"+tasknew.scheduledExecutionTime()); try { Thread.sleep(500); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } class CustomTimerTask extends TimerTask { @Override public void run() { System.out.println("working on"); } }
Output
Let us compile and run the above program, this will produce the following result.
Time is :1675410583664 working on working on working on working on working on working on
To Continue Learning Please Login